设计一个三维向量类,并实现向量的加法、减法以及向量与标量的乘法和除法运算(要求重载__add__、__sub__、__mul__等方法)
时间: 2023-06-05 19:05:17 浏览: 58
好的,这是一个关于编程的问题,下面是我的回答:
先定义一个三维向量类:
```python
class Vector3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
```
接下来实现向量的加法、减法以及向量与标量的乘法和除法运算:
```python
class Vector3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, scalar):
return Vector3D(self.x * scalar, self.y * scalar, self.z * scalar)
def __truediv__(self, scalar):
return Vector3D(self.x / scalar, self.y / scalar, self.z / scalar)
```
这样,我们就可以用类似于下面的方式对三维向量进行加、减、乘、除运算:
```python
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
# 向量加法
v3 = v1 + v2
print(v3.x, v3.y, v3.z) # 输出:5 7 9
# 向量减法
v3 = v1 - v2
print(v3.x, v3.y, v3.z) # 输出:-3 -3 -3
# 向量与标量的乘法
v3 = v1 * 2
print(v3.x, v3.y, v3.z) # 输出:2 4 6
# 向量与标量的除法
v3 = v1 / 2
print(v3.x, v3.y, v3.z) # 输出:0.5 1.0 1.5
```
希望我的回答能够解决你的问题。如果你有更多的问题,请随时提出。
阅读全文
相关推荐
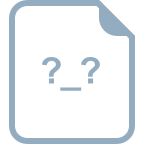









