#include <stdio.h>#include <string.h>#include <stdlib.h>#define MAX_SMS_LENGTH 200#define MAX_NAME_LENGTH 8#define MAX_PHONE_LENGTH 11typedef struct { char word[MAX_SMS_LENGTH]; /*短信内容*/ int length; /*短信长度*/} Message;typedef struct { char name[MAX_NAME_LENGTH]; /*姓名*/ char phone[MAX_PHONE_LENGTH]; /*电话号码或手机号码*/} Note;void extract_phone_number(Message* message, Note* note) { int start = 0; int phone_start = -1; int phone_end = -1; while (start < message->length) { if (message->word[start] >= '0' && message->word[start] <= '9') { phone_start = start; phone_end = start; start++; while (start < message->length && message->word[start] >= '0' && message->word[start] <= '9') { phone_end = start; start++; } if (phone_end - phone_start == 6 || phone_end - phone_start == 7) { strncpy(note->phone, message->word + phone_start, phone_end - phone_start + 1); note->phone[phone_end - phone_start + 1] = '\0'; } else if (phone_end - phone_start == 10 || phone_end - phone_start == 11) { strncpy(note->phone, message->word + phone_start, phone_end - phone_start + 1); note->phone[phone_end - phone_start + 1] = '\0'; } if (strlen(note->phone) > 0) { break; } } else { start++; } }}void save_to_contact(Note* note) { printf("请输入姓名:"); fgets(note->name, MAX_NAME_LENGTH, stdin); note->name[strlen(note->name) - 1] = '\0'; /*将最后的换行符去掉*/ printf("已将 %s 的电话号码 %s 存储到通讯录中。\n", note->name, note->phone); /*将 note 存储到通讯录中*/}int main() { Message message; Note note; char input[MAX_SMS_LENGTH + 1]; /*输入缓存*/ // 读取短信 printf("请输入一条短信:\n"); fgets(input, MAX_SMS_LENGTH + 1, stdin); message.length = strlen(input) - 1; strncpy(message.word, input, message.length); message.word[message.length] = '\0'; memset(note.name, 0, MAX_NAME_LENGTH); memset(note.phone, 0, MAX_PHONE_LENGTH); extract_phone_number(&message, ¬e); save_to_contact(¬e); return 0;}怎么读取信息
时间: 2024-04-28 17:20:53 浏览: 70
这个代码实现了一个简单的从输入中提取电话号码并存储到通讯录的功能。具体流程如下:
1. 定义了两个结构体 Message 和 Note,分别表示短信和联系人信息。
2. 实现了一个函数 extract_phone_number,该函数接收一个 Message 指针和一个 Note 指针,从 Message 结构体中提取出电话号码,并将其存储到 Note 结构体中。
3. 实现了一个函数 save_to_contact,该函数接收一个 Note 指针,从用户输入中读取联系人姓名,并将姓名和电话号码存储到通讯录中。
4. 在主函数中,首先读取用户输入的短信内容,然后调用 extract_phone_number 和 save_to_contact 函数分别提取电话号码并存储到通讯录中。
如果您有具体的问题或需求,请提出来,我会尽力帮助您。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdbool.h> #define MAX_CONTACTS 100 #define PASSWORD "1234" 解释
这段代码包含了四个预处理指令(`#define`),以及四个头文件`<stdio.h>`, `<stdlib.h>`, `<string.h>`, 和 `<stdbool.h>`。其中:
1. `#define MAX_CONTACTS 100`: 宏定义了一个常量`MAX_CONTACTS`,它的值为100。这通常用于设置某个数据结构的最大容量,如数组或动态分配内存的上限。
2. `#define PASSWORD "1234"`: 宏定义了一个字符串常量`PASSWORD`,其值为"1234"。这可能是某个程序中用于验证用户密码的一个默认口令。
3. `#include <stdio.h>`: 引入了标准输入输出库,提供基本的I/O操作功能,如printf和scanf等。
4. `#include <stdlib.h>`: 引入了标准C库,包含内存管理函数,如malloc、calloc、realloc和free等。
5. `#include <string.h>`: 提供字符串处理函数,如strlen, strcpy, strcmp等。
6. `#include <stdbool.h>`: 引入布尔类型(bool)的支持,使得代码可以更清晰地表达条件判断。
这段代码可能会在处理用户输入、验证密码或者其他涉及字符串和内存管理的场景中发挥作用。
#include+<stdio.h> #include+<stdlib.h> #include<string.h> #define+MAX_NAME+5 #define+MAX_VERTEX_NUM
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME 5
#define MAX_VERTEX_NUM 40
int main() {
// Your code here
return 0;
}
```
这是一个简单的C程序的框架,包含了头文件`stdio.h`、`stdlib.h`和`string.h`,以及定义了常量`MAX_NAME`和`MAX_VERTEX_NUM`。你可以在`// Your code here`的位置编写你的具体代码逻辑。这个程序的作用是创建一个空的C程序框架,你可以在其中添加你需要的功能。
阅读全文
相关推荐
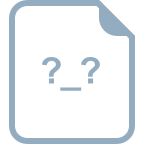
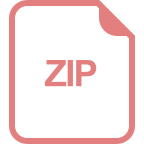
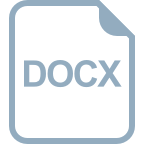



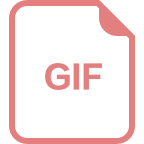
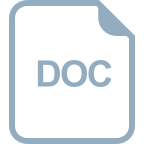
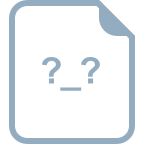
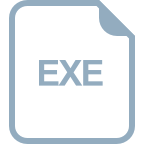



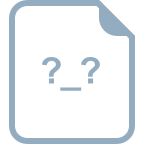
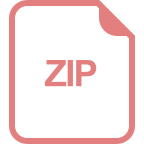