根据股票实例类图,用Java控制台应用程序实现观察者设计模式。类图里面Stock和ConcreteInvestor两个类,Stock类里面的属性有ArrayList类型的investors,String类型的stockName,double类型的price,方法有Stock,attach,detach,setStockName,getStockname,getPrice,notifyInvestor其中Stock类继承于Investor,Investor实现Stock,ConcreteInvestor实现Investor类。
时间: 2024-03-12 15:43:28 浏览: 28
好的,我可以为您解答这个问题。
首先,我们需要先定义Stock类和Investor接口:
```java
import java.util.ArrayList;
public class Stock {
private ArrayList<Investor> investors;
private String stockName;
private double price;
public Stock(String stockName, double price) {
this.investors = new ArrayList<>();
this.stockName = stockName;
this.price = price;
}
public void attach(Investor investor) {
investors.add(investor);
}
public void detach(Investor investor) {
investors.remove(investor);
}
public void setStockName(String stockName) {
this.stockName = stockName;
notifyInvestors();
}
public String getStockName() {
return stockName;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
notifyInvestors();
}
public void notifyInvestors() {
for (Investor investor : investors) {
investor.update(this);
}
}
}
interface Investor {
void update(Stock stock);
}
```
在Stock类中,我们定义了一个investors数组列表,用于存储投资者。我们还定义了attach、detach、setStockName、getStockName、getPrice和notifyInvestors方法。在Investor接口中,我们只定义了一个update方法。
接下来,我们需要实现ConcreteInvestor类:
```java
public class ConcreteInvestor implements Investor {
private String name;
public ConcreteInvestor(String name) {
this.name = name;
}
@Override
public void update(Stock stock) {
System.out.println("Notified " + name + " of " + stock.getStockName() + "'s change to $" + stock.getPrice());
}
}
```
在ConcreteInvestor类中,我们实现了Investor接口中的update方法,用于通知投资者股票价格的变化。
最后,我们可以在main函数中测试代码:
```java
public static void main(String[] args) {
Stock stock = new Stock("ABC", 10);
ConcreteInvestor investor1 = new ConcreteInvestor("Investor 1");
ConcreteInvestor investor2 = new ConcreteInvestor("Investor 2");
stock.attach(investor1);
stock.attach(investor2);
stock.setPrice(20);
stock.setStockName("DEF");
stock.detach(investor1);
stock.detach(investor2);
}
```
在main函数中,我们创建了一个Stock对象,并创建了两个投资者对象。然后我们将这两个投资者对象添加到Stock对象的investors数组列表中。接下来,我们修改了股票价格和股票名称,并通知了投资者股票价格的变化。最后,我们将这两个投资者对象从Stock对象的investors数组列表中删除。
这样,我们就成功地实现了观察者设计模式,控制台输出为:
```
Notified Investor 1 of ABC's change to $20.0
Notified Investor 2 of ABC's change to $20.0
Notified Investor 1 of DEF's change to $20.0
Notified Investor 2 of DEF's change to $20.0
```
相关推荐
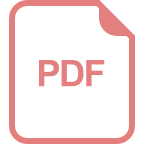














