jdbc.batch_size: 100
时间: 2024-05-26 10:08:04 浏览: 121
jdbc.batch_size是一个JDBC的配置参数,它指定了在批处理操作中,一次向数据库提交的最大SQL语句数量。例如,当jdbc.batch_size设置为100时,每当向批处理中添加100条SQL语句时,这些SQL语句就会一次性提交到数据库中执行。
这个参数的设置可以大大提高批量操作的效率,因为它减少了网络通信和数据库执行的次数。但是需要注意的是,如果一次性提交的SQL语句数量太多,可能会导致数据库缓存溢出等问题。因此,需要根据实际情况合理地设置jdbc.batch_size参数。
相关问题
package com.zhazhapan.efo.dao.sqlprovider; import com.zhazhapan.efo.EfoApplication; import com.zhazhapan.efo.modules.constant.ConfigConsts; import com.zhazhapan.util.Checker; import org.apache.ibatis.annotations.Param; import org.apache.ibatis.jdbc.SQL; /** * @author admin */ public class AuthSqlProvider { public String updateAuthById() { return CommonSqlProvider.updateAuthById("auth"); } public String batchDelete(@Param("ids") String ids) { return "delete from auth where id in " + (ids.startsWith("(") ? "" : "(") + ids + (ids.endsWith(")") ? "" : ")"); } public String getAuthBy(@Param("id") long id, @Param("userId") int userId, @Param("fileId") long fileId, @Param ("fileName") String fileName, @Param("offset") int offset) { String sql = new SQL() {{ SELECT("a.id,a.user_id,a.file_id,u.username,f.name file_name,f.local_url,a.is_downloadable,a" + "" + "" + ".is_uploadable,a.is_deletable,a.is_updatable,a.is_visible,a.create_time"); FROM("auth a"); JOIN("user u on u.id=a.user_id"); JOIN("file f on f.id=a.file_id"); if (id > 0) { WHERE("a.id=#{id}"); } if (userId > 0) { WHERE("u.id=#{userId}"); } if (fileId > 0) { WHERE("f.id=#{fileId}"); } else if (Checker.isNotEmpty(fileName)) { WHERE("f.local_url like '%" + fileName + "%'"); } ORDER_BY("a." + EfoApplication.settings.getStringUseEval(ConfigConsts.AUTH_ORDER_BY_OF_SETTINGS)); }}.toString(); int size = EfoApplication.settings.getIntegerUseEval(ConfigConsts.AUTH_PAGE_SIZE_OF_SETTINGS); return sql + " limit " + (offset * size) + "," + size; } } 请用你的专业知识帮我详细解释以上代码: 1.具体实现了什么功能 2、具体用到什么知识点
这是一个Java文件,包名为com.zhazhapan.efo.dao.sqlprovider,其中使用了一些类库,如Apache Mybatis等。这个文件定义了AuthSqlProvider类,里面有一些方法,如updateAuthById、batchDelete和getAuthBy等,用于提供SQL语句。其中getAuthBy方法使用了SQL类和SELECT、FROM和JOIN等方法,构建出一个查询数据库的SQL语句,用于获取包含一些特定条件的Auth信息。
1.保存为文件:可以将获取到的数据保存为文本文件、JSON文件、XML文件等格式的文件。保存为文本文件可以使用Java IO流进行操作,保存为JSON或XML文件可以使用相关的Java库,如Jackson或JAXB。 2.保存到数据库:可以将获取到的数据保存到关系型数据库或非关系型数据库中。关系型数据库可以使用Java JDBC进行操作,非关系型数据库可以使用Java驱动程序或者ORM框架进行操作。 3.保存到缓存:可以将获取到的数据保存到缓存中,如Redis、Memcached等。可以使用Java客户端库进行操作。 4.保存到消息队列:可以将获取到的数据保存到消息队列中,如Kafka、RabbitMQ等。可以使用Java客户端库进行操作。请将这几种方法明细化 给出相应的实例代码及相关操作步骤
1. 保存为文件
可以将获取到的数据保存为文本文件、JSON文件、XML文件等格式的文件。保存为文本文件可以使用Java IO流进行操作,保存为JSON或XML文件可以使用相关的Java库,如Jackson或JAXB。
Java IO流保存为文本文件示例代码:
```java
String data = "Hello World";
File file = new File("data.txt");
try (FileWriter writer = new FileWriter(file)) {
writer.write(data);
} catch (IOException e) {
e.printStackTrace();
}
```
Jackson库保存为JSON文件示例代码:
```java
ObjectMapper mapper = new ObjectMapper();
Map<String, Object> dataMap = new HashMap<>();
dataMap.put("name", "John");
dataMap.put("age", 30);
File file = new File("data.json");
try {
mapper.writeValue(file, dataMap);
} catch (IOException e) {
e.printStackTrace();
}
```
2. 保存到数据库
可以将获取到的数据保存到关系型数据库或非关系型数据库中。关系型数据库可以使用Java JDBC进行操作,非关系型数据库可以使用Java驱动程序或者ORM框架进行操作。
Java JDBC保存到关系型数据库示例代码:
```java
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, username, password)) {
String sql = "INSERT INTO users (name, age) VALUES (?, ?)";
PreparedStatement statement = conn.prepareStatement(sql);
statement.setString(1, "John");
statement.setInt(2, 30);
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
```
MongoDB保存到非关系型数据库示例代码:
```java
String uri = "mongodb://localhost:27017";
try (MongoClient client = MongoClients.create(uri)) {
MongoDatabase database = client.getDatabase("mydatabase");
MongoCollection<Document> collection = database.getCollection("users");
Document document = new Document("name", "John")
.append("age", 30);
collection.insertOne(document);
} catch (MongoException e) {
e.printStackTrace();
}
```
3. 保存到缓存
可以将获取到的数据保存到缓存中,如Redis、Memcached等。可以使用Java客户端库进行操作。
Redis保存到缓存示例代码:
```java
Jedis jedis = new Jedis("localhost");
jedis.set("name", "John");
jedis.expire("name", 60);
```
Memcached保存到缓存示例代码:
```java
MemcachedClient client = new MemcachedClient(new InetSocketAddress("localhost", 11211));
client.set("name", 60, "John");
```
4. 保存到消息队列
可以将获取到的数据保存到消息队列中,如Kafka、RabbitMQ等。可以使用Java客户端库进行操作。
Kafka保存到消息队列示例代码:
```java
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("acks", "all");
props.put("retries", 0);
props.put("batch.size", 16384);
props.put("linger.ms", 1);
props.put("buffer.memory", 33554432);
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
ProducerRecord<String, String> record = new ProducerRecord<>("mytopic", "name", "John");
producer.send(record);
producer.close();
```
RabbitMQ保存到消息队列示例代码:
```java
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare("myqueue", false, false, false, null);
String message = "Hello World";
channel.basicPublish("", "myqueue", null, message.getBytes());
} catch (IOException | TimeoutException e) {
e.printStackTrace();
}
```
以上示例代码仅供参考,实际应用中需要根据具体情况进行修改。
阅读全文
相关推荐
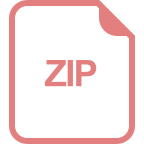
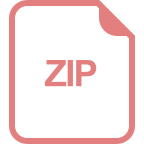
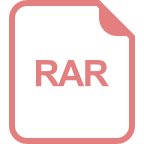
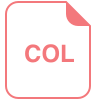



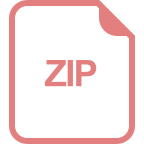
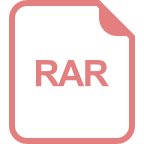
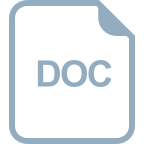
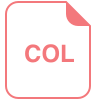
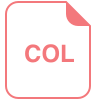
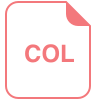
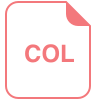
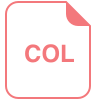

