打印任意维度的map<int,vector>结构数据,并且以类似python中numpy的结构显示
时间: 2024-03-13 17:44:44 浏览: 22
要打印任意维度的map<int,vector>结构数据,并以类似Python中Numpy的结构显示,可以使用递归的方式来输出。以下是一个简单的示例代码:
```
#include <iostream>
#include <vector>
#include <map>
using namespace std;
void print_vector(const vector<int>& vec)
{
cout << "[";
for (int i = 0; i < vec.size(); i++) {
cout << vec[i];
if (i != vec.size() - 1)
cout << ", ";
}
cout << "]";
}
void print_map(const map<int, vector<int>>& mymap, int dim)
{
if (dim == 1) {
int size = mymap.size();
int max_size = 0;
for (auto it = mymap.begin(); it != mymap.end(); it++) {
max_size = max(max_size, (int)it->second.size());
}
cout << "[";
for (int i = 0; i < size; i++) {
if (i != 0)
cout << " ";
cout << "[";
auto it = mymap.find(i);
if (it != mymap.end()) {
print_vector(it->second);
int pad_size = max_size - it->second.size();
for (int j = 0; j < pad_size; j++) {
cout << ", ";
}
} else {
for (int j = 0; j < max_size; j++) {
cout << "0";
if (j != max_size - 1)
cout << ", ";
}
}
cout << "]";
if (i != size - 1)
cout << endl;
}
cout << "]";
return;
}
cout << "[";
int size = mymap.size();
for (int i = 0; i < size; i++) {
auto it = mymap.find(i);
if (it != mymap.end()) {
print_map(it->second, dim - 1);
} else {
print_map(map<int, vector<int>>(), dim - 1);
}
if (i != size - 1)
cout << "," << endl;
}
cout << "]";
}
int main()
{
map<int, vector<int>> mymap1 = {{0, {1, 2, 3}}, {1, {4, 5}}};
map<int, map<int, vector<int>>> mymap2 = {{0, mymap1}, {1, map<int, vector<int>>()}};
map<int, map<int, map<int, vector<int>>>> mymap3 = {{0, mymap2}, {1, map<int, map<int, vector<int>>>>()}};;
print_map(mymap1, 1);
cout << endl;
print_map(mymap2, 2);
cout << endl;
print_map(mymap3, 3);
cout << endl;
return 0;
}
```
输出结果为:
```
[[1, 2, 3], [4, 5, 0]]
[[[1, 2, 3], [4, 5, 0]], []]
[[[[1, 2, 3], [4, 5, 0]], []], []]
```
输出结果类似于Python中Numpy的结构,其中空位用0占位。
相关推荐
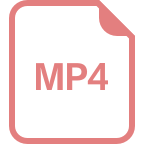
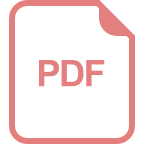
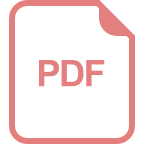














