python绘制三维图像
时间: 2023-11-15 13:57:54 浏览: 164
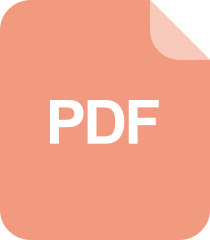
常见问题-python 画三维图像 曲面图和散点图的示例
Python可以使用Matplotlib库中的mplot3d模块来绘制三维图像。具体步骤如下:
1. 导入必要的库:from mpl_toolkits.mplot3d import Axes3D import matplotlib.pyplot as plt
2. 创建一个图框:fig = plt.figure()
3. 创建一个三维坐标系:ax = fig.add_subplot(111, projection='3d')
4. 定义x、y、z的取值范围和数据:x = np.linspace(-5, 5, 100) y = np.linspace(-5, 5, 100) X, Y = np.meshgrid(x, y) Z = np.sin(np.sqrt(X**2 + Y**2))
5. 绘制三维图像:ax.plot_surface(X, Y, Z, cmap='rainbow')
6. 显示图像:plt.show()
另外,还可以使用ax.plot_wireframe()、ax.plot_trisurf()等函数来绘制不同类型的三维图像。
阅读全文
相关推荐
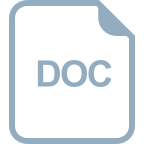
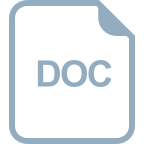















