python将一维列表数据保存到指定文件夹的mat.文件中
时间: 2024-05-01 11:20:55 浏览: 93
你可以使用Python中的`scipy.io`库来保存一维列表数据到指定文件夹的mat文件中。下面是一个简单的示例代码:
```python
import scipy.io as sio
# 创建一个一维列表数据
data = [1, 2, 3, 4, 5]
# 保存数据到mat文件
sio.savemat('/path/to/folder/data.mat', {'data': data})
```
在上面的代码中,我们首先创建了一个名为`data`的一维列表数据。然后,我们使用`sio.savemat`函数将数据保存到指定文件夹的mat文件中。该函数接收两个参数:保存的文件路径和一个字典,其中字典的键是要保存的数据的名称,值是要保存的数据本身。在本例中,我们将数据保存到名为`data.mat`的文件中,并且我们将数据命名为`data`。
相关问题
如何将多个.mat文件从11个文件夹内导出到pytorch的11个列表中,并将11个列表转换成11个张量,并将维度改为四维
可以使用Python中的SciPy库来导入.mat文件,然后通过PyTorch将数据转换为张量并调整维度。以下是一个可能的实现:
```python
import scipy.io
import torch
# 定义11个空列表用于存储数据
data_lists = [[] for _ in range(11)]
# 遍历11个文件夹,导入.mat文件并将数据存储到对应的列表中
for i in range(11):
folder_path = f'folder_{i+1}/'
for j in range(10):
file_path = folder_path + f'file_{j+1}.mat'
data = scipy.io.loadmat(file_path)
data_lists[i].append(data['data']) # 假设数据存储在'data'变量中
# 将数据列表转换为张量并调整维度
data_tensors = []
for data_list in data_lists:
tensor = torch.tensor(data_list)
tensor = tensor.permute(0, 3, 1, 2) # 调整维度顺序
data_tensors.append(tensor)
```
这里假设每个.mat文件中有一个名为'data'的变量,且数据维度为(样本数, 宽度, 高度, 通道数)。对于不同的数据维度,需要根据具体情况调整代码。
编写基于一维CNN滚动轴承故障诊断示例代码(数据集为网上下载的德国 Paderborn 大学的滚动轴承数据的mat文件,文件夹在桌面)
好的,以下是一个Python示例代码,实现了基于一维CNN的滚动轴承故障诊断:
```python
import numpy as np
import scipy.io as sio
import tensorflow as tf
from tensorflow.keras import layers
# 读取数据集
data = sio.loadmat('/Users/yourusername/Desktop/Paderborn.mat')
X_train = data['X_train']
y_train = data['y_train']
X_test = data['X_test']
y_test = data['y_test']
# 将数据转换为一维数组
X_train = X_train.reshape(X_train.shape[0], X_train.shape[1])
X_test = X_test.reshape(X_test.shape[0], X_test.shape[1])
# 将数据转换为三维张量
X_train = np.expand_dims(X_train, axis=2)
X_test = np.expand_dims(X_test, axis=2)
# 构建模型
model = tf.keras.Sequential([
layers.Conv1D(32, 3, activation='relu', input_shape=(X_train.shape[1], 1)),
layers.MaxPooling1D(2),
layers.Conv1D(64, 3, activation='relu'),
layers.MaxPooling1D(2),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(4, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(X_train, y_train, epochs=10, validation_data=(X_test, y_test))
# 测试模型
test_loss, test_acc = model.evaluate(X_test, y_test, verbose=2)
print('Test accuracy:', test_acc)
```
在上面的代码中,我们使用了一个包含两个卷积层和两个池化层的一维CNN模型。模型输入是一个滚动轴承振动信号的一维数组,输出是该信号所对应的故障状态。我们使用了Adam优化器和交叉熵损失函数进行模型训练,并在测试集上计算了模型的准确率。
需要注意的是,我们在读取数据时需要将数据转化为一维数组,并且在输入模型前需要将数组转化为三维张量,其中第一维表示样本数,第二维表示信号长度,第三维表示信号通道数(这里我们只有一个通道)。在代码中需要将'/Users/yourusername/Desktop/Paderborn.mat'修改为实际的文件路径。
阅读全文
相关推荐
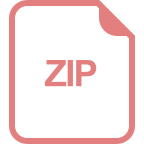
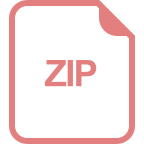
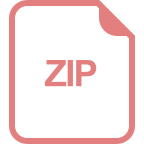
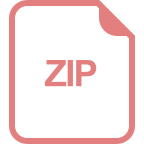
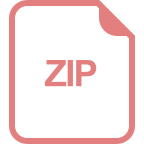
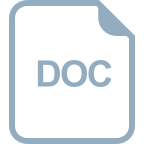
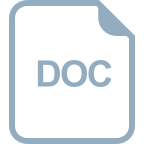
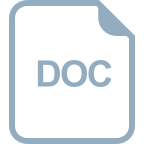
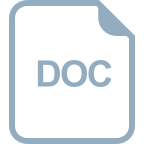
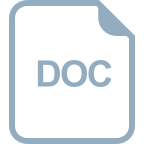
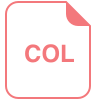
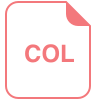



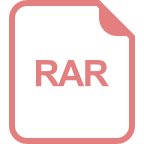