利用队列在c语言中输出杨辉三角
时间: 2024-09-30 09:03:52 浏览: 63
在C语言中,杨辉三角是一个经典的算法问题,通常通过动态数组来构建,因为它的每一行都是前一行和自身加和的结果。我们可以利用队列来存储当前行的数据,并按顺序生成下一行。以下是使用队列实现杨辉三角的一种方法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_ROWS 50
// 队列结构体
typedef struct {
int* array;
size_t size;
size_t top;
} Queue;
// 初始化队列
Queue init_queue(int rows) {
Queue q;
q.array = (int*)malloc(sizeof(int) * (rows + 1));
q.size = rows + 1;
q.top = -1;
return q;
}
// 入队操作
void enqueue(Queue& q, int value) {
if (q.top == q.size - 1)
q.top++;
q.array[q.top + 1] = value;
q.top++;
}
// 出队操作
int dequeue(Queue& q) {
if (q.top == -1)
return 0;
q.top--;
return q.array[q.top];
}
// 打印杨辉三角
void print_pascal_triangle(Queue q) {
while (!queue_empty(q)) {
for (size_t i = 0; i <= q.top; ++i) {
printf("%d ", q.array[i]);
}
printf("\n");
dequeue(q);
}
}
// 清空队列
bool queue_empty(const Queue& q) {
return q.top == -1;
}
int main() {
int rows = 5;
Queue triangle_q = init_queue(rows);
// 构建并打印杨辉三角
for (int i = 0; i < rows; ++i) {
for (int j = 0; j <= i; ++j) {
enqueue(triangle_q, factorial(i) / (factorial(j) * factorial(i - j)));
}
}
print_pascal_triangle(triangle_q);
free(triangle_q.array); // 释放内存
return 0;
}
// 计算阶乘
int factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
阅读全文
相关推荐
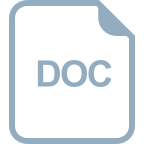
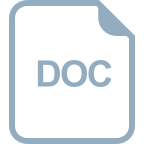
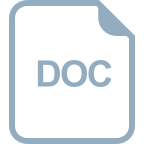








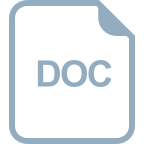






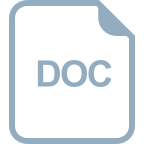