java获取图片旋转度_java实现图片角度旋转并获得图片信息
时间: 2023-09-19 12:05:52 浏览: 299
要获取图片的旋转度并实现图片角度旋转,可以使用Java的ImageIO和AffineTransform类。以下是一个示例代码:
```java
import java.awt.geom.AffineTransform;
import java.awt.image.AffineTransformOp;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class ImageRotateExample {
public static void main(String[] args) throws Exception {
// 读取图片文件
File input = new File("input.png");
BufferedImage image = ImageIO.read(input);
// 获取图片旋转度
int angle = getRotateAngle(image);
System.out.println("图片旋转度:" + angle);
// 旋转图片
BufferedImage rotatedImage = rotateImage(image, angle);
// 保存旋转后的图片
File output = new File("output.png");
ImageIO.write(rotatedImage, "png", output);
}
// 获取图片旋转度
public static int getRotateAngle(BufferedImage image) {
// 获取图片的宽度和高度
int width = image.getWidth();
int height = image.getHeight();
// 获取图片的四个角的像素值
int topLeft = image.getRGB(0, 0);
int topRight = image.getRGB(width-1, 0);
int bottomLeft = image.getRGB(0, height-1);
int bottomRight = image.getRGB(width-1, height-1);
// 判断图片是否旋转
if (topLeft == topRight && topRight == bottomLeft && bottomLeft == bottomRight) {
return 0;
}
else if (topLeft == bottomRight && topRight == bottomLeft) {
return 180;
}
else if (topLeft == topRight) {
return -90;
}
else {
return 90;
}
}
// 旋转图片
public static BufferedImage rotateImage(BufferedImage image, int angle) {
// 计算旋转中心点
int centerX = image.getWidth() / 2;
int centerY = image.getHeight() / 2;
// 创建变换矩阵
AffineTransform transform = new AffineTransform();
transform.rotate(Math.toRadians(angle), centerX, centerY);
// 创建变换操作对象
AffineTransformOp op = new AffineTransformOp(transform, AffineTransformOp.TYPE_BILINEAR);
// 执行变换操作
return op.filter(image, null);
}
}
```
在上面的示例代码中,getRotateAngle方法用于获取图片的旋转度,rotateImage方法用于旋转图片。这两个方法都使用了AffineTransform类来进行变换操作。注意:以上代码只能处理图片旋转角度为0、90、180、270度的情况。如果需要处理其他旋转角度的图片,需要进行更复杂的处理。
阅读全文
相关推荐








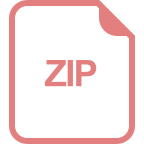








