使用【python】写【文本相似度分析】的代码如何让僵尸速度更快
时间: 2024-10-04 14:01:38 浏览: 32
在Python中,要提高文本相似度分析的速度,可以采用一些策略和优化技巧。例如:
1. **预处理**:对输入的文本进行标准化,如去除停用词、标点符号和数字,降低词汇量并转换成小写。使用`nltk`库中的`stopwords`模块可以帮助完成这个步骤。
```python
from nltk.corpus import stopwords
import string
def preprocess(text):
stop_words = set(stopwords.words('english'))
text = ' '.join([word.lower() for word in text.split() if word.isalpha() and word not in stop_words])
return text.translate(str.maketrans('', '', string.punctuation))
```
2. **分块处理**:如果文本非常长,可以考虑分块计算相似度,而不是一次性加载整个文本。比如,使用`minibatch_generator`函数来迭代处理。
```python
def minibatch_generator(data, batch_size):
data_len = len(data)
for i in range(0, data_len, batch_size):
yield data[i:i + batch_size]
# 用法示例
text1 = ... # 长文本1
texts = ... # 列表形式的待比较文本
batched_texts = list(minibatch_generator(texts, batch_size=1000))
for batch in batched_texts:
# 计算每批文本之间的相似度
similarities = compute_similarity(preprocess(text1), [preprocess(text) for text in batch])
```
3. **使用高效的相似度算法**:选择适合大规模文本的数据结构和算法,如TF-IDF(Term Frequency-Inverse Document Frequency)或者基于向量的模型(如Word2Vec、BERT)。`sklearn`库中的`TfidfVectorizer`和`cosine_similarity`可以提供高效支持。
4. **并行计算**:利用Python的多线程或多进程库(如`concurrent.futures`),将任务分解到不同的处理器核心上,加速计算。
5. **硬件加速**:如果可能,可以考虑使用GPU加速,比如使用`spaCy`等库结合CUDA进行计算。
```python
import spacy
nlp_gpu = spacy.load('en_core_web_sm', disable=['parser', 'ner'], device='cuda')
```
通过以上优化,可以显著提高文本相似度分析的效率。但是具体的性能提升取决于数据规模、原始代码质量和可用资源。
阅读全文
相关推荐
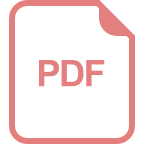
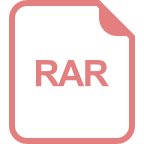















