prime算法数据结构
时间: 2023-08-15 07:05:39 浏览: 193
Prime算法是一种用于寻找图中最小生成树的算法,它是基于贪心思想的。在Prime算法中,我们从一个起始顶点开始,逐步构建最小生成树,每次选择一个与当前最小生成树连接的边且权值最小的顶点加入最小生成树中,直到将所有的顶点都加入到最小生成树中为止。
在实现Prime算法时,通常需要借助于一些数据结构来辅助操作。常用的数据结构有以下两种:
1. 优先队列(Priority Queue):优先队列用于维护当前与最小生成树连接的边中权值最小的边。通过优先队列,我们可以快速获得权值最小的边并将其加入到最小生成树中。常见的实现方式有二叉堆和斐波那契堆。
2. 标记数组(Marked Array):标记数组用于标记已经加入到最小生成树中的顶点,以避免重复加入。在每次选择边时,我们可以通过标记数组来判断顶点是否已经被加入到最小生成树中。通常使用布尔类型的数组来实现。
这两种数据结构在Prime算法的实现中起到了重要的作用,能够提高算法的效率和准确性。通过结合优先队列和标记数组,我们可以高效地找到图中的最小生成树。
相关问题
prime算法C语言
prime算法是一种用于寻找素数的常见算法。下面是一个使用C语言实现的简单示例代码:
```c
#include <stdio.h>
int isPrime(int num) {
if (num <= 1) {
return 0;
}
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) {
return 0;
}
}
return 1;
}
int main() {
int n;
printf("请输入一个正整数: ");
scanf("%d", &n);
if (isPrime(n)) {
printf("%d 是素数\n", n);
} else {
printf("%d 不是素数\n", n);
}
return 0;
}
```
这个程序中,我们定义了一个`isPrime`函数,用于判断一个数是否为素数。然后在`main`函数中,我们读取用户输入的正整数,并调用`isPrime`函数来判断该数是否为素数。最后根据判断结果输出相应的消息。
请记住,这只是一个简单的示例,可能不适用于处理非常大的数。在实际应用中,可能需要使用更高效的算法和数据结构来处理大数素数判断。
prime算法和迪杰斯特拉算法区别
### Prim算法与Dijkstra算法的区别
#### 应用场景
Prim算法主要用于求解最小生成树问题,即在一个加权无向图中找到一棵包含所有顶点的子树,并使得这棵树上所有边的权重之和尽可能小。而Dijkstra算法则用于计算单源最短路径问题,在给定起点的情况下找出到达其他各节点的最短距离[^1]。
#### 实现方式
在实现过程中,两种算法都采用了贪心策略来逐步构建最终的结果集。然而,对于每一步的选择标准有所不同:
- **Prim算法**:每次从未处理过的结点集合中选取一个离当前已加入到生成树中的最近的一个新结点作为扩展对象;
- **Dijkstra算法**:则是基于已经确定的距离值,挑选出尚未访问过且具有最小临时标记(估计距离)的下一个目标进行更新操作。
```python
# Python示例代码展示两者的区别
import heapq
def prim(graph, start):
mst = []
visited = set([start])
edges = [(cost, start, to) for to, cost in graph[start].items()]
heapq.heapify(edges)
while edges:
(cost, frm, to) = heapq.heappop(edges)
if to not in visited:
visited.add(to)
mst.append((frm, to, cost))
for next_node, weight in graph[to].items():
if next_node not in visited:
heapq.heappush(edges, (weight, to, next_node))
return mst
def dijkstra(graph, start):
shortest_paths = {start: (None, 0)}
current_node = start
visited = set()
while True:
visited.add(current_node)
destinations = graph[current_node].keys()
weights_to_current_node = graph[current_node]
for next_node in destinations:
weight = weights_to_current_node[next_node] + shortest_paths[current_node][1]
if next_node not in shortest_paths or weight < shortest_paths[next_node][1]:
shortest_paths[next_node] = (current_node, weight)
unvisited_nodes = dict((node, path[1]) for node, path in shortest_paths.items() if node not in visited)
if not unvisited_nodes:
break
current_node = min(unvisited_nodes, key=unvisited_nodes.get)
return shortest_paths
```
#### 时间复杂度
关于时间效率方面,当采用二叉堆优化时,
- **Prim算法** 的平均情况下的时间复杂度可以达到 \(O(E\log V)\),这里\(E\)代表边数,\(V\)表示顶点数目;
- 对于 **Dijkstra算法**, 如果使用简单的数组结构,则其时间复杂度为 \(O(V^2)\)[^2]。但如果同样利用优先队列优化的话,也可以降低至接近线性的水平,具体取决于数据结构的选择以及输入规模特性。
阅读全文
相关推荐




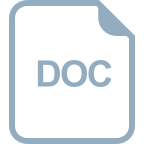







