如何用C语言编写一个程序来验证输入的数学表达式(包含运算数和加减乘除以及括号)的语法并计算其值?输入应为单行字符串,如果表达式有效,输出计算结果;若无效,则给出相应的错误提示。请提供详细的输入输出格式说明和示例代码。
时间: 2024-12-22 15:30:11 浏览: 3
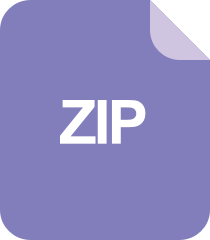
C语言实现计算器简单混合运算

在C语言中,编写这样一个程序可以使用词法分析和解析技术。这里我们可以简单地采用递归下降解析法(Recursive Descent Parsing),不过这涉及到复杂的语法处理,不适合在这里详细解释。下面是一个简单的例子,这个版本只能处理加减运算,并且没有考虑括号、乘除运算以及更复杂的情况:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAX_TOKEN_LENGTH 50
typedef struct {
char type;
double value;
} Token;
Token get_token(char *str) {
Token token;
int i = 0;
while (isspace(str[i])) ++i; // skip whitespace
if (isdigit(str[i])) { // digit found
token.type = 'NUM';
token.value = atof(&str[i]);
while (isdigit(str[i + 1])) ++i;
} else if (str[i] == '+') { // operator found
token.type = '+';
token.value = 0;
++i;
} else if (str[i] == '-') { // operator found
token.type = '-';
token.value = 0;
++i;
} else {
printf("Invalid token: %s\n", str);
exit(1); // error handling
}
token.value += atof(&str[i]); // add any remaining digits to the value
return token;
}
double evaluate_expression(const char *expr) {
Token token;
double result = 0;
while (*expr != '\0') {
token = get_token(expr);
if (token.type == 'NUM') {
result += token.value;
} else if (token.type == '+' || token.type == '-') {
result += token.value;
} else {
printf("Unexpected operator: %c\n", token.type);
exit(1);
}
expr += strlen(token.type) + 1; // advance to next token
}
return result;
}
int main() {
char input[100];
printf("Enter a mathematical expression (e.g., 1 + 2): ");
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // remove newline character at end
double result = evaluate_expression(input);
printf("The calculated value is: %.2f\n", result);
return 0;
}
```
**输入输出格式**:
1. 输入:`Enter a mathematical expression (e.g., 1 + 2):`
2. 输出示例:`The calculated value is: 3.00`
注意这个例子非常基础,实际的解析器需要处理更多情况,如括号、优先级、乘除运算等。此外,它也不适合用于生产环境,因为它的错误处理并不完善,只是为了演示基本原理。如果你需要一个完整的解决方案,建议使用已有的库,比如开源的tokenizer和parser库,例如ANTLR或Flex/Bison。
阅读全文
相关推荐
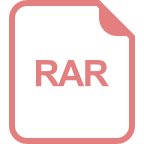
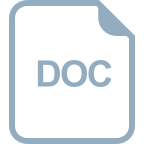





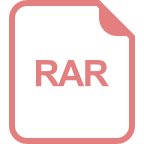
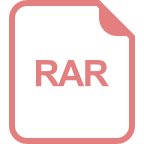
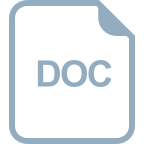
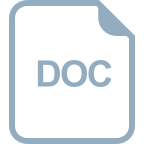
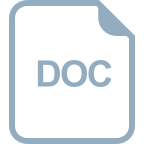
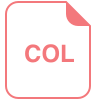
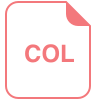


