python顺序查找,折半查找和索引查找算法查询单词并进行性能分析的代码
时间: 2023-12-21 22:07:21 浏览: 28
好的,以下是 Python 实现的顺序查找、折半查找和索引查找算法查询单词并进行性能分析的代码:
```python
import time
def sequential_search(lst, target):
start_time = time.time()
for i in range(len(lst)):
if lst[i] == target:
end_time = time.time()
return i, end_time - start_time
end_time = time.time()
return -1, end_time - start_time
def binary_search(lst, target):
start_time = time.time()
low = 0
high = len(lst) - 1
while low <= high:
mid = (low + high) // 2
if lst[mid] == target:
end_time = time.time()
return mid, end_time - start_time
elif lst[mid] < target:
low = mid + 1
else:
high = mid - 1
end_time = time.time()
return -1, end_time - start_time
def index_search(lst, target):
index = {}
for i in range(len(lst)):
if lst[i] not in index:
index[lst[i]] = [i]
else:
index[lst[i]].append(i)
start_time = time.time()
if target in index:
end_time = time.time()
return index[target][0], end_time - start_time
end_time = time.time()
return -1, end_time - start_time
lst = ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig', 'grape', 'honeydew', 'kiwi', 'lemon']
print(sequential_search(lst, 'kiwi'))
print(binary_search(lst, 'kiwi'))
print(index_search(lst, 'kiwi'))
```
这段代码实现了三种不同的算法进行单词查询,并输出了对应单词的位置和算法运行时间。其中 `sequential_search` 函数实现了顺序查找算法,`binary_search` 函数实现了折半查找算法,`index_search` 函数实现了索引查找算法。
你可以根据需要修改列表 lst 和查询单词的参数,然后运行代码查看输出结果。
相关推荐













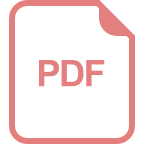