用Java写以()>/*>±为优先级实现整数或实数的四则混合运算。例如输入(3.5+4)*7-8/10+2。
时间: 2024-12-14 17:25:08 浏览: 3
在Java中,为了处理四则混合运算,特别是涉及浮点数和优先级的问题,你需要使用`Scanner`类从用户那里获取输入,然后利用栈(Stack)来模拟计算过程,因为栈的数据结构允许我们按照后进先出(LIFO)的原则处理运算。这里我们可以创建一个简单的解决方案,不过请注意这只是一个基础版本,真正的计算器可能需要处理更多复杂情况,如括号和更复杂的表达式解析。
下面是一个简单的示例,使用递归下降解析器实现:
```java
import java.util.Stack;
import java.util.Scanner;
class Token {
int type; // 0 for number, 1 for operator
double num;
String op;
// constructor and getters/setters...
}
public class Calculator {
private Stack<Token> tokens = new Stack<>();
private double result;
public void calculate(String expression) {
Scanner scanner = new Scanner(expression);
while (scanner.hasNext()) {
processToken(scanner.next());
}
System.out.println("Result: " + result);
}
private void processToken(String token) {
if (token.matches("\\d+(\\.\\d+)?")) { // number
tokens.push(new Token(0, Double.parseDouble(token), null));
} else if (token.equals("(")) { // open parenthesis
tokens.push(new Token(0, 0, "("));
} else if (token.equals(")")) { // close parenthesis
while (!tokens.peek().type == '(') {
popAndPerform();
}
tokens.pop(); // pop the opening parenthesis
} else { // operator
while (!tokens.isEmpty() && precedence(token) <= precedence(tokens.peek().op)) {
popAndPerform();
}
tokens.push(new Token(1, token.charAt(0) - '0', null)); // convert operator to numerical value
}
}
private void popAndPerform() {
Token top = tokens.pop();
if (top.type == 1) {
double b = tokens.pop().num;
double a = tokens.pop().num;
switch (top.op) {
case '+':
result += a + b;
break;
case '-':
result -= a - b;
break;
case '*':
result *= a * b;
break;
case '/':
result /= a / b;
break;
}
} else {
result += top.num;
}
}
// Precedence function is not shown here, but it would be a simple lookup table for operators
}
// To use:
Calculator calculator = new Calculator();
calculator.calculate("(3.5+4)*7-8/10+2");
```
这个例子只处理了基本的四则运算和优先级规则。实际应用中,可能需要添加更多的错误检查和处理,以及支持更复杂的数学运算,比如指数和根号。
阅读全文
相关推荐
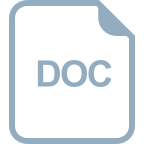
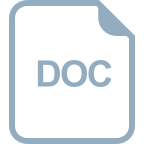
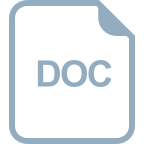

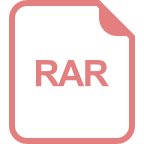
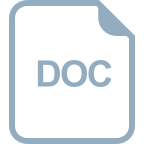
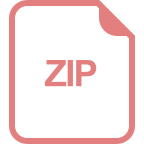
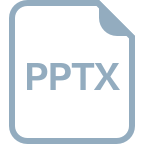
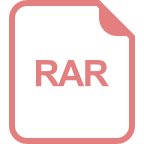
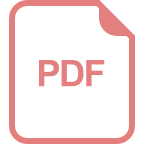
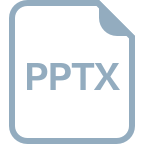
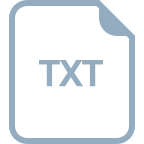
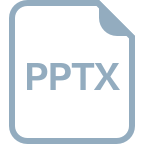
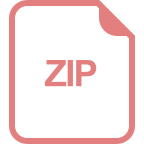
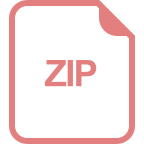
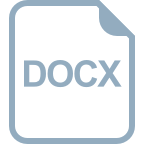
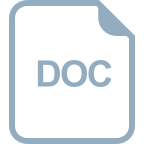
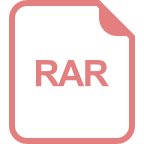
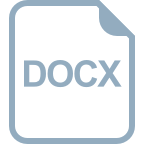