私钥sm2解密 c++
时间: 2023-08-16 22:08:30 浏览: 75
要在 C++ 中解密 SM2 密钥对的私钥,你可以使用 OpenSSL 库提供的函数和工具。下面是一个使用 OpenSSL 解密 SM2 私钥的示例代码:
```cpp
#include <iostream>
#include <openssl/evp.h>
#include <openssl/pem.h>
#include <openssl/ec.h>
#include <openssl/err.h>
void handleErrors()
{
unsigned long errCode;
char errBuff[256];
while ((errCode = ERR_get_error()) != 0)
{
ERR_error_string_n(errCode, errBuff, sizeof(errBuff));
std::cerr << "OpenSSL error: " << errBuff << std::endl;
}
throw std::runtime_error("OpenSSL error");
}
EC_KEY *loadPrivateKey(const std::string &privateKeyPath)
{
FILE *fp = fopen(privateKeyPath.c_str(), "rb");
if (fp == nullptr)
{
std::cerr << "Failed to open private key file" << std::endl;
return nullptr;
}
EC_KEY *ecKey = PEM_read_ECPrivateKey(fp, nullptr, nullptr, nullptr);
if (ecKey == nullptr)
{
std::cerr << "Failed to read private key" << std::endl;
fclose(fp);
return nullptr;
}
fclose(fp);
return ecKey;
}
int main()
{
const std::string privateKeyPath = "/path/to/private_key.pem";
OpenSSL_add_all_algorithms();
ERR_load_crypto_strings();
EC_KEY *privateKey = loadPrivateKey(privateKeyPath);
if (privateKey == nullptr)
{
handleErrors();
return 1;
}
// 输出解密后的私钥信息
const BIGNUM *privateKeyBN = EC_KEY_get0_private_key(privateKey);
char *privateKeyHex = BN_bn2hex(privateKeyBN);
std::cout << "Decrypted private key: " << privateKeyHex << std::endl;
OPENSSL_free(privateKeyHex);
EC_KEY_free(privateKey);
EVP_cleanup();
ERR_free_strings();
return 0;
}
```
在示例代码中,我们定义了 `loadPrivateKey` 函数,用于加载包含加密的 SM2 私钥的 PEM 文件。然后,我们使用 `PEM_read_ECPrivateKey` 函数读取并解密私钥。
请确保将 `privateKeyPath` 替换为你实际的私钥文件路径。
编译并运行示例代码,将会输出解密后的 SM2 私钥信息。请注意,你需要在编译时链接 OpenSSL 库,方法请参考前面的回答。
如果你遇到问题或错误,请确保 OpenSSL 库版本正确,并检查私钥文件是否正确。
相关推荐
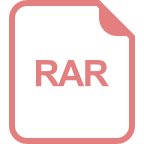














