java将学生数据用io存入文件s,若文件不存在则创建再存入
时间: 2024-03-04 14:51:52 浏览: 20
可以使用以下代码将学生数据存入文件中:
```java
import java.io.*;
public class StudentIOExample {
public static void main(String[] args) throws IOException {
File file = new File("s.txt"); // 创建文件对象
if (!file.exists()) { // 如果文件不存在,则创建文件
file.createNewFile();
}
// 将学生数据写入文件中
Student s1 = new Student("张三", 20, "男");
Student s2 = new Student("李四", 21, "女");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(file));
oos.writeObject(s1);
oos.writeObject(s2);
oos.close();
// 从文件中读取学生数据
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file));
try {
while (true) {
Student s = (Student) ois.readObject();
System.out.println(s);
}
} catch (EOFException e) {
// 文件读取完毕
}
ois.close();
}
static class Student implements Serializable {
String name;
int age;
String gender;
public Student(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
public String toString() {
return "姓名:" + name + ",年龄:" + age + ",性别:" + gender;
}
}
}
```
在上面的代码中,我们定义了一个 `Student` 类表示学生,该类实现了 `Serializable` 接口,以便可以将其对象序列化并写入文件中。
接下来,我们创建一个 `File` 对象表示要操作的文件(这里是 `s.txt`),并检查该文件是否存在。如果文件不存在,则调用 `createNewFile()` 方法创建该文件。
然后,我们创建两个 `Student` 对象,并使用 `ObjectOutputStream` 将这两个对象写入文件中。`ObjectOutputStream` 可以将任意对象序列化并写入文件中。
最后,我们使用 `ObjectInputStream` 从文件中读取学生数据,并输出到控制台中。注意,这里的文件读取操作不需要显式地检查文件是否存在,因为如果文件不存在,会直接抛出异常。当读取到文件末尾时,`ObjectInputStream` 会抛出 `EOFException` 异常,我们可以通过捕获该异常来判断文件是否已经读取完毕。
相关推荐
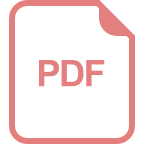
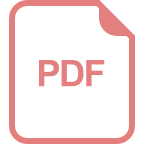
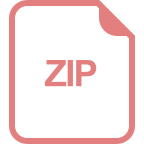














