//#include <iostream> //#include <cstring> //using namespace std; //struct node //{ // int date; // node* next; //}; //void creat_list(node*& head,int n) //{ // head = new node; // head->next = NULL; // for (int i = 0; i <= n; i++) // { // node* p = new node; // p->date = i; // p->next = head->next; // head->next = p; // } //} //void pop_list(node* head) //{ // node* p = head->next; // while (p->next != NULL) // { // cout << p->date<<'\n'; // p = p->next; // } //} //void main() //{ // node* head; // creat_list(head, 5); // pop_list(head); //}给我讲讲这段代码
时间: 2023-07-04 22:30:04 浏览: 103
这段代码定义了一个单向链表的结构体 `node`,其中包含一个整型数据 `date` 和一个指向下一个节点的指针 `next`。然后定义了两个函数,分别是 `creat_list` 和 `pop_list`。
`creat_list` 函数用于创建链表,接受两个参数,一个是指向头节点的指针 `head`,另一个是链表的长度 `n`。在函数中,先创建一个头节点,并将其 `next` 指针初始化为 `NULL`。然后循环创建 `n` 个节点,每个节点的数据域 `date` 赋值为 `i`,并将其插入到链表的头部。
`pop_list` 函数用于遍历链表并输出每个节点的数据域 `date`。在函数中,先定义一个指针变量 `p` 指向头节点的下一个节点,然后循环输出 `p` 的数据域 `date`,直到遍历完整个链表。
最后在 `main` 函数中,先定义一个指向头节点的指针 `head`,然后调用 `creat_list` 函数创建一个长度为 5 的链表,并调用 `pop_list` 函数遍历并输出链表中的每个节点的数据。
相关问题
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
解释一下//#include <iostream> //#include <cstring> //using namespace std; //struct node //{ // int date; // node* next; //}; //void creat_list(node*& head,int n) //{ // head = new node; // head->next = NULL; // for (int i = 0; i <= n; i++) // { // node* p = new node; // p->date = i; // p->next = head->next; // head->next = p; // } //} //void pop_list(node* head) //{ // node* p = head->next; // while (p->next != NULL) // { // cout << p->date<<'\n'; // p = p->next; // } //} //void main() //{ // node* head; // creat_list(head, 5); // pop_list(head); //}
这段代码实现了一个简单的链表数据结构。首先定义了一个结构体`node`,包含一个`int`类型的数据`date`和一个指向下一个节点的指针`next`。然后定义了一个函数`creat_list`,通过输入参数`n`创建一个长度为`n`的链表,并将链表头结点的指针赋给`head`。在`creat_list`函数中,首先创建头结点,然后循环创建`n`个结点,将它们依次插入到链表的头部。最后定义了一个函数`pop_list`,遍历链表并输出每个结点的数据。在`main`函数中,创建了一个头结点`head`,并调用`creat_list`函数创建长度为`5`的链表,然后调用`pop_list`函数遍历并输出链表中的每个结点的数据。
阅读全文
相关推荐
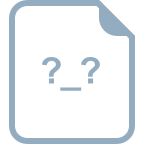
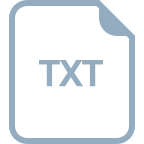













