用Python写个微信客户端给指定好友发送消息的模板
时间: 2023-08-31 16:43:21 浏览: 229
### 回答1:
可以参考下面这个示例:import itchat itchat.login() #登陆微信 friends = itchat.search_friends(name='xxx') #搜索指定好友 userName = friends[0]['UserName'] #获取好友的UserName itchat.send('Hello, 我是Python程序发出的消息', toUserName=userName) #发送消息
### 回答2:
使用Python编写微信客户端给指定好友发送消息的模板,可以使用itchat库实现。
首先,我们需要安装itchat库。可以使用pip命令在终端中执行以下命令安装:
```python
pip install itchat
```
安装完成后,我们可以在Python代码中引入itchat库:
```python
import itchat
```
然后,我们需要使用itchat库登录微信账号,可以调用login()函数进行登录:
```python
itchat.login()
```
在登录成功后,可以使用search_friends()函数来查找指定好友的信息。该函数需要传入好友的微信昵称,返回一个字典,包含了好友的详细信息。我们可以通过该字典获取到好友的微信id,然后使用send_msg()函数向好友发送消息:
```python
friend = itchat.search_friends(nickname='好友昵称')
friend_id = friend[0]['UserName']
itchat.send_msg('要发送的消息', friend_id)
```
最后,我们需要调用itchat.run()函数来运行微信客户端。该函数会一直运行,直到我们主动退出程序。
完整的代码如下:
```python
import itchat
itchat.login()
friend = itchat.search_friends(nickname='好友昵称')
friend_id = friend[0]['UserName']
itchat.send_msg('要发送的消息', friend_id)
itchat.run()
```
运行以上代码,我们就可以实现使用Python编写微信客户端给指定好友发送消息的功能了。
需要注意的是,该模板只能在登录的设备上运行,不支持后台运行。
### 回答3:
下面是一个使用Python编写的微信客户端模板,可以用来给指定好友发送消息。
```python
import itchat
# 登录微信账号
itchat.auto_login()
# 获取好友列表
friend_list = itchat.get_friends()
# 找到指定好友的UserName
target_friend_name = "指定好友的昵称"
target_friend_username = None
for friend in friend_list:
if friend["NickName"] == target_friend_name:
target_friend_username = friend["UserName"]
break
# 如果找不到指定好友,打印错误信息并退出
if target_friend_username is None:
print("找不到指定好友")
exit()
# 发送消息
message = "你好,这是一条测试消息"
itchat.send(message, toUserName=target_friend_username)
# 登出微信账号
itchat.logout()
```
请注意,运行该脚本之前需要安装itchat模块。可以使用以下命令安装:
```shell
pip install itchat
```
其中,`指定好友的昵称`需要替换为你要发送消息的好友的昵称。你也可以根据其他信息进行筛选,比如备注名等。
该脚本登陆微信账号后,会先获取好友列表,然后通过遍历好友列表找到指定好友的UserName。如果找不到,则打印错误信息并退出。发送消息时,只需调用`itchat.send`函数,将消息内容和目标好友的UserName传入即可。
注意,该脚本只能在有图形界面的系统上运行,因为itchat模块需要扫描二维码进行登录。如果需要在无界面的服务器上运行,可以考虑使用itchat的可靠登陆方式,具体可以参考itchat的文档。
阅读全文
相关推荐
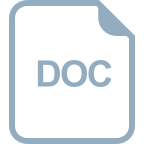
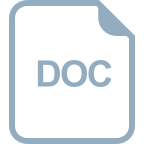
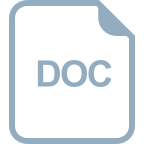
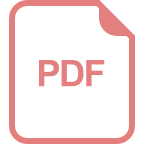
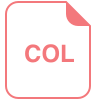
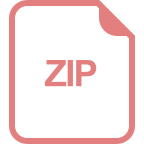
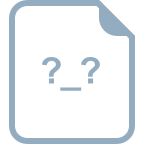
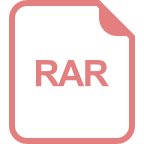
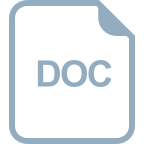
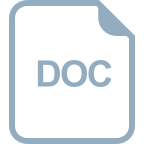
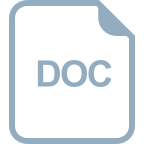
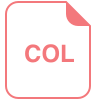
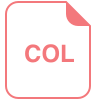
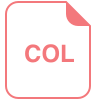
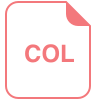
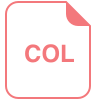
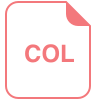
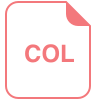
