ring_buffer_queue调用
时间: 2023-12-25 13:04:58 浏览: 40
Ring buffer queue(环形缓冲队列)是一种数据结构,常用于实现多线程之间的数据传输。它的特点是可以在固定大小的存储空间中存储数据,并且支持高效的读写操作。
下面是一个简单的 ring buffer queue 的 C++ 实现,供参考:
```cpp
#include <iostream>
#include <mutex>
#include <condition_variable>
template<typename T>
class RingBufferQueue {
public:
RingBufferQueue(size_t capacity) : buffer_(capacity), capacity_(capacity) {}
void push(const T& value) {
std::unique_lock<std::mutex> lock(mutex_);
while (size_ == capacity_) {
not_full_cv_.wait(lock);
}
buffer_[tail_] = value;
tail_ = (tail_ + 1) % capacity_;
++size_;
not_empty_cv_.notify_one();
}
T pop() {
std::unique_lock<std::mutex> lock(mutex_);
while (size_ == 0) {
not_empty_cv_.wait(lock);
}
T value = buffer_[head_];
head_ = (head_ + 1) % capacity_;
--size_;
not_full_cv_.notify_one();
return value;
}
private:
std::vector<T> buffer_;
size_t capacity_;
size_t head_ = 0;
size_t tail_ = 0;
size_t size_ = 0;
std::mutex mutex_;
std::condition_variable not_full_cv_;
std::condition_variable not_empty_cv_;
};
```
使用该 ring buffer queue,可以在多线程中进行数据传输。例如,可以在一个线程中 push 数据,而在另一个线程中 pop 数据:
```cpp
RingBufferQueue<int> queue(10);
void producer() {
for (int i = 0; i < 100; ++i) {
queue.push(i);
}
}
void consumer() {
for (int i = 0; i < 100; ++i) {
int value = queue.pop();
std::cout << value << std::endl;
}
}
int main() {
std::thread t1(producer);
std::thread t2(consumer);
t1.join();
t2.join();
return 0;
}
```
在该示例中,producer 线程不断向 queue 中 push 数据,而 consumer 线程则不断从 queue 中 pop 数据。由于 queue 的容量为 10,因此当 queue 中的数据达到 10 时,producer 线程会被阻塞,直到 consumer 线程 pop 出数据,使得 queue 中的数据量小于 10 时才会继续 push 数据;同样地,当 queue 中的数据为 0 时,consumer 线程会被阻塞,直到 producer 线程 push 数据,使得 queue 中的数据量大于 0 时才会继续 pop 数据。这样,就可以保证在多线程情况下,数据的传输是安全和高效的。
相关推荐
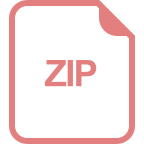














