用python将蚁群算法中蚂蚁的路径可视化出来
时间: 2024-02-28 16:56:11 浏览: 29
以下是一个简单的例子,其中我们使用matplotlib库来绘制图形:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成一个二维迷宫地图
maze = np.array([
[0, 1, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 1, 0],
[1, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
])
# 定义一个Ant类
class Ant:
def __init__(self, x, y):
self.x = x
self.y = y
self.path = [(x, y)] # 记录蚂蚁走过的路径
def move(self, maze):
# ...
# 这里省略了蚂蚁移动的代码
# ...
# 记录蚂蚁的路径
self.path.append((self.x, self.y))
# 初始化蚂蚁集合
ants = [Ant(2, 4), Ant(2, 4), Ant(2, 4)]
# 绘制迷宫地图
fig, ax = plt.subplots()
for i in range(maze.shape[0]):
for j in range(maze.shape[1]):
if maze[i,j] == 1:
ax.fill([j, j, j+1, j+1], [maze.shape[0]-i-1, maze.shape[0]-i, maze.shape[0]-i, maze.shape[0]-i-1], 'k')
ax.set_xlim([0, maze.shape[1]])
ax.set_ylim([0, maze.shape[0]])
# 绘制蚂蚁的路径
colors = ['r', 'g', 'b'] # 不同蚂蚁使用不同的颜色
for i, ant in enumerate(ants):
x = [pos[0] for pos in ant.path]
y = [pos[1] for pos in ant.path]
ax.plot(x, y, colors[i % len(colors)])
plt.show()
```
该代码会生成一个迷宫地图,并在图中绘制蚂蚁的路径。你可以根据自己的需求来修改迷宫地图和蚂蚁的路径。
相关推荐
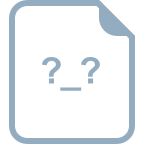
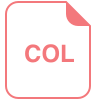
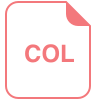

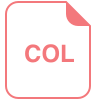
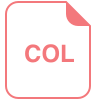









