用C语言写一个STM32硬件i2c程序
时间: 2023-08-14 14:06:02 浏览: 56
下面是一个简单的STM32硬件I2C程序,使用了STM32F103C8T6开发板和Keil MDK编译器:
```c
#include "stm32f10x.h"
#define I2C1_OWN_ADDR 0xA0
#define I2C_SLAVE_ADDR 0x50
void I2C1_init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
I2C_InitTypeDef I2C_InitStruct;
// Enable clock for GPIOB and I2C1
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
// Configure PB6 and PB7 as I2C1_SCL and I2C1_SDA
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_Init(GPIOB, &GPIO_InitStruct);
// Configure I2C1
I2C_InitStruct.I2C_Mode = I2C_Mode_I2C;
I2C_InitStruct.I2C_DutyCycle = I2C_DutyCycle_2;
I2C_InitStruct.I2C_OwnAddress1 = I2C1_OWN_ADDR;
I2C_InitStruct.I2C_Ack = I2C_Ack_Enable;
I2C_InitStruct.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit;
I2C_InitStruct.I2C_ClockSpeed = 100000;
I2C_Init(I2C1, &I2C_InitStruct);
// Enable I2C1
I2C_Cmd(I2C1, ENABLE);
}
void I2C1_start(void)
{
// Send start condition
I2C_GenerateSTART(I2C1, ENABLE);
// Wait until start condition is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT));
}
void I2C1_stop(void)
{
// Send stop condition
I2C_GenerateSTOP(I2C1, ENABLE);
// Wait until stop condition is sent
while (I2C_GetFlagStatus(I2C1, I2C_FLAG_STOPF));
}
void I2C1_write(uint8_t data)
{
// Send data byte
I2C_SendData(I2C1, data);
// Wait until data byte is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
}
uint8_t I2C1_read_ack(void)
{
// Enable ACK after receiving data byte
I2C_AcknowledgeConfig(I2C1, ENABLE);
// Wait until data byte is received
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_RECEIVED));
// Read data byte
uint8_t data = I2C_ReceiveData(I2C1);
return data;
}
uint8_t I2C1_read_nack(void)
{
// Disable ACK after receiving last data byte
I2C_AcknowledgeConfig(I2C1, DISABLE);
// Wait until data byte is received
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_RECEIVED));
// Read data byte
uint8_t data = I2C_ReceiveData(I2C1);
return data;
}
int main(void)
{
uint8_t data[] = "Hello world!";
uint8_t buffer[50];
// Initialize I2C1
I2C1_init();
// Send start condition
I2C1_start();
// Send slave address and write bit
I2C1_write(I2C_SLAVE_ADDR << 1 | 0);
// Send data
for (int i = 0; i < sizeof(data); i++) {
I2C1_write(data[i]);
}
// Send stop condition
I2C1_stop();
// Wait for a while
for (int i = 0; i < 1000000; i++);
// Send start condition
I2C1_start();
// Send slave address and read bit
I2C1_write(I2C_SLAVE_ADDR << 1 | 1);
// Read data
for (int i = 0; i < sizeof(data) - 1; i++) {
buffer[i] = I2C1_read_ack();
}
// Read last data byte with NACK
buffer[sizeof(data) - 1] = I2C1_read_nack();
// Send stop condition
I2C1_stop();
// Print received data
printf("%s", buffer);
while (1);
}
```
该程序用于向I2C从设备发送"Hello world!"字符串,然后从该设备读取相同的字符串并将其打印到串口。
相关推荐
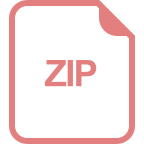
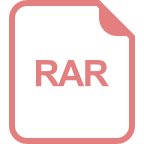














