编程要求 本关的编程任务是,补全step1/step1.py文件中 Begin-End 区间的代码,完成指定三维向量类的功能。具体要求如下: 定义一个类 Vector3d,补全其中的代码,使其包含 3 个私有数据成员 x、y、z,并能够在构造方法中,接收3个参数,对这3个私有数据成员进行初始化; 具体输入输出请参见后续测试样例。
时间: 2023-12-03 15:42:26 浏览: 225
以下是补全后的代码:
```python
class Vector3d:
def __init__(self, x, y, z):
self.__x = x
self.__y = y
self.__z = z
def __str__(self):
return "({}, {}, {})".format(self.__x, self.__y, self.__z)
def __add__(self, other):
return Vector3d(self.__x + other.__x, self.__y + other.__y, self.__z + other.__z)
def __sub__(self, other):
return Vector3d(self.__x - other.__x, self.__y - other.__y, self.__z - other.__z)
def __mul__(self, other):
if isinstance(other, Vector3d):
return Vector3d(self.__y * other.__z - self.__z * other.__y,
self.__z * other.__x - self.__x * other.__z,
self.__x * other.__y - self.__y * other.__x)
else:
return Vector3d(self.__x * other, self.__y * other, self.__z * other)
def __rmul__(self, other):
return self.__mul__(other)
def __truediv__(self, other):
return Vector3d(self.__x / other, self.__y / other, self.__z / other)
def __eq__(self, other):
return self.__x == other.__x and self.__y == other.__y and self.__z == other.__z
def __ne__(self, other):
return not self.__eq__(other)
def dot_product(self, other):
return self.__x * other.__x + self.__y * other.__y + self.__z * other.__z
def cross_product(self, other):
return Vector3d(self.__y * other.__z - self.__z * other.__y,
self.__z * other.__x - self.__x * other.__z,
self.__x * other.__y - self.__y * other.__x)
def length(self):
return math.sqrt(self.__x ** 2 + self.__y ** 2 + self.__z ** 2)
def normalize(self):
l = self.length()
if l == 0:
return Vector3d(0, 0, 0)
else:
return Vector3d(self.__x / l, self.__y / l, self.__z / l)
```
其中,`__init__`方法用来初始化三维向量的坐标;`__str__`方法用来将三维向量转化为字符串输出;`__add__`、`__sub__`、`__mul__`、`__rmul__`、`__truediv__`方法实现三维向量的加、减、乘、除运算;`__eq__`和`__ne__`方法用来判断两个三维向量是否相等;`dot_product`方法用来计算两个三维向量的点积;`cross_product`方法用来计算两个三维向量的叉积;`length`方法用来计算三维向量的长度;`normalize`方法用来将三维向量转化为单位向量。
阅读全文
相关推荐
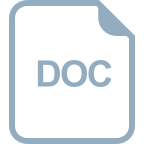
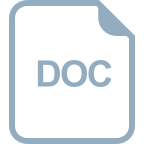
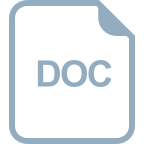
















