如何使得Curator注册的MySQL连接节点中存储的是实际的连接对象或连接状态,curator版本为curator-recipes5.0.0,Java版本为18,MySQL版本为8.0.21.给出完整实现代码。
时间: 2023-07-16 11:12:07 浏览: 46
Curator提供了一个名为ConnectionStateManager的接口,它可以用于管理连接状态。我们可以使用它来实现在Curator注册的MySQL连接节点中存储实际的连接对象或连接状态。
以下是一个基本的实现代码:
```java
import org.apache.curator.framework.CuratorFramework;
import org.apache.curator.framework.recipes.cache.ChildData;
import org.apache.curator.framework.recipes.cache.NodeCache;
import org.apache.curator.framework.recipes.cache.NodeCacheListener;
import org.apache.curator.framework.recipes.nodes.PersistentNode;
import org.apache.curator.framework.state.ConnectionState;
import org.apache.curator.framework.state.ConnectionStateListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnectionStateManager implements ConnectionStateListener, NodeCacheListener {
private final CuratorFramework curatorFramework;
private final String nodePath;
private final String connectionString;
private final String username;
private final String password;
private final NodeCache nodeCache;
private Connection connection;
public MySQLConnectionStateManager(CuratorFramework curatorFramework, String nodePath, String connectionString, String username, String password) {
this.curatorFramework = curatorFramework;
this.nodePath = nodePath;
this.connectionString = connectionString;
this.username = username;
this.password = password;
this.nodeCache = new NodeCache(curatorFramework, nodePath);
}
public void start() throws Exception {
// 创建持久节点
PersistentNode persistentNode = new PersistentNode(curatorFramework, PersistentNode.Mode.EPHEMERAL, nodePath);
persistentNode.start();
// 添加连接状态监听器
curatorFramework.getConnectionStateListenable().addListener(this);
// 添加节点数据监听器
nodeCache.getListenable().addListener(this);
// 启动节点数据监听器
nodeCache.start();
// 初始化连接
connection = createConnection(nodeCache.getCurrentData());
}
public void close() throws IOException {
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
// 处理关闭连接时发生的异常
}
}
nodeCache.close();
}
private Connection createConnection(ChildData childData) throws SQLException {
if (childData == null) {
return null;
}
String connectionString = new String(childData.getData());
return DriverManager.getConnection(connectionString, username, password);
}
@Override
public void stateChanged(CuratorFramework client, ConnectionState newState) {
switch (newState) {
case CONNECTED:
case RECONNECTED:
try {
// 连接恢复,重新创建连接对象
connection = createConnection(nodeCache.getCurrentData());
} catch (SQLException e) {
// 处理连接创建时发生的异常
}
break;
case SUSPENDED:
case LOST:
// 连接中断或丢失,关闭连接对象
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
// 处理关闭连接时发生的异常
}
connection = null;
}
break;
default:
break;
}
}
@Override
public void nodeChanged() throws Exception {
// 节点数据变化,重新创建连接对象
connection = createConnection(nodeCache.getCurrentData());
}
}
```
使用示例:
```java
CuratorFramework curatorFramework = ...; // 创建CuratorFramework实例
String nodePath = "/mysql/connection"; // MySQL连接节点路径
String connectionString = "jdbc:mysql://localhost:3306/mydb"; // MySQL连接字符串
String username = "root"; // MySQL用户名
String password = "password"; // MySQL密码
MySQLConnectionStateManager connectionStateManager = new MySQLConnectionStateManager(curatorFramework, nodePath, connectionString, username, password);
connectionStateManager.start(); // 启动连接状态管理器
// ...使用连接对象进行数据库操作
connectionStateManager.close(); // 关闭连接状态管理器
```
相关推荐
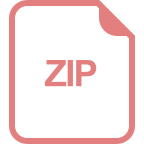
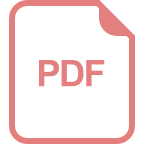
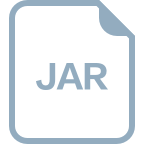














