Python中如何提取某一列字符串中的中文
时间: 2024-09-15 19:10:39 浏览: 79
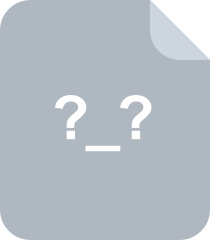
python 提取文字段落中的日期字符串

在Python中,如果你有一个包含中文的DataFrame或列表,并想从某一列提取中文字符串,你可以使用Pandas库。首先,确保你已经安装了pandas和pyhanlp等用于处理中文的库。下面是一个基本的例子:
```python
import pandas as pd
from pyhanlp import *
# 假设你有一个名为df的DataFrame,其中有一列叫'chinese_column'
df = pd.DataFrame({
'chinese_column': ['你好', '世界', '我爱你']
})
# 使用正则表达式提取中文字符,HanLP可以用于更精确的分词和分析
def extract_chinese(text):
chinese_pattern = re.compile(r'[^\u4e00-\u9fa5]')
return [char for char in text if not chinese_pattern.match(char)]
# 应用到DataFrame的特定列
df['extracted_chinese'] = df['chinese_column'].apply(extract_chinese)
# 打印结果
print(df)
```
在这个例子中,`extract_chinese`函数会过滤出非中文字符。然而,如果需要更复杂的中文文本处理,如分词、词性标注等,可以考虑使用HanLP库进行操作。
阅读全文
相关推荐
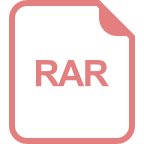
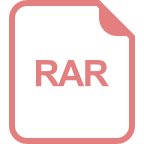















