用C语言实现elgamal完整代码
时间: 2024-12-11 15:28:43 浏览: 35
ElGamal是一种非对称加密算法,用于公钥密码学。以下是C语言实现ElGamal加密和解密的简化版本,这里仅提供核心部分,完整的代码会包含更多错误检查、用户输入处理等:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// ElGamal公钥和私钥结构体
typedef struct {
uint32_t p; // 模素
uint32_t g; // 群生成元
uint32_t y; // 公钥
uint32_t x; // 私钥
} KeyPair;
// 随机数生成
uint32_t random(uint32_t n) {
return rand() % n;
}
// 计算模幂运算
uint32_t power(uint32_t base, uint32_t exponent, uint32_t modulus) {
if (exponent == 0) return 1;
if (exponent % 2 == 0) return power(base * base % modulus, exponent / 2, modulus);
return base * power(base, exponent - 1, modulus) % modulus;
}
// ElGamal加密函数
void encrypt(KeyPair* public_key, uint32_t m, uint32_t* ciphertext) {
uint32_t k = random(public_key->p - 1); // 选择随机指数
uint32_t r = power(public_key->g, k, public_key->p); // 生成第一部分
uint32_t c1 = (m * power(r, public_key->x, public_key->p)) % public_key->p; // 加密消息
ciphertext[0] = r; // 存储第一部分
ciphertext[1] = c1; // 存储第二部分
}
// ElGamal解密函数
uint32_t decrypt(KeyPair* private_key, const uint32_t* ciphertext) {
uint32_t r = ciphertext[0];
uint32_t c1 = ciphertext[1];
uint32_t s = power(r, private_key->p - 2, private_key->p); // 计算解密指数
return power(c1, s, private_key->p); // 解密消息
}
int main() {
srand(time(0)); // 初始化随机种子
// 生成密钥对
KeyPair key_pair;
key_pair.p = ...; // 设置大质数
key_pair.g = ...; // 设置群生成元
key_pair.x = ...; // 用户私钥,注意这是保密信息,不会在这里显示
// 假设用户输入明文m
uint32_t plaintext = ...;
// 加密
uint32_t ciphertext[2];
encrypt(&key_pair, plaintext, ciphertext);
// 输出加密结果,通常这部分会被隐藏
printf("Encrypted message: (%u, %u)\n", ciphertext[0], ciphertext[1]);
// 解密
uint32_t decrypted_text = decrypt(&key_pair, ciphertext);
printf("Decrypted text: %u\n", decrypted_text);
return 0;
}
```
请注意,上述代码仅为示例,实际应用中你需要确保安全性,如设置足够大的质数、保护私钥安全等。此外,由于篇幅限制,这里未包含错误处理和用户交互的部分。
阅读全文
相关推荐
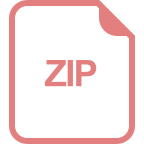
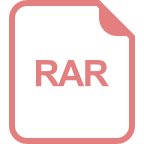






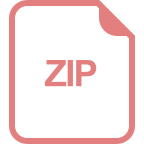
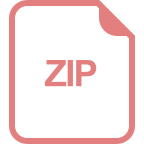
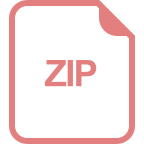
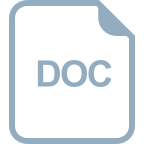
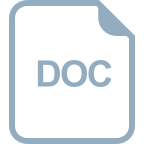
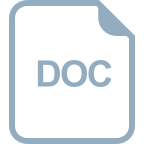
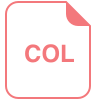


