unity三维小球游戏制作
时间: 2023-09-04 20:16:03 浏览: 189
制作unity三维小球游戏,可以按照以下步骤:
1.创建一个新的Unity项目,选择3D模板。
2.导入小球模型,可以从网上下载或者自己制作。
3.添加物理引擎,将小球添加到场景中,并添加一个球体碰撞体。
4.创建地形,可以使用Unity自带的地形工具或者导入外部地形。
5.添加障碍物,可以在场景中添加一些障碍物,如墙壁、坑洞等等。
6.添加相机,设置相机视角。
7.添加光源,设置场景的光照效果。
8.添加音效,可以为小球的滚动、碰撞等添加音效。
9.编写脚本,实现小球的移动、碰撞检测、计分等游戏逻辑。
10.发布游戏,将游戏发布到目标平台上。
相关问题
unity三维小球游戏代码
以下是一个简单的Unity三维小球游戏的代码示例:
```csharp
using UnityEngine;
using System.Collections;
public class BallController : MonoBehaviour {
public float speed; // 球体移动速度
private Rigidbody rb;
void Start () {
rb = GetComponent<Rigidbody>();
}
void FixedUpdate () {
// 获取用户输入
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
// 根据用户输入移动球体
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
rb.AddForce(movement * speed);
}
void OnTriggerEnter(Collider other) {
if (other.gameObject.CompareTag("PickUp")) {
other.gameObject.SetActive(false);
}
}
}
```
这段代码实现了用户控制球体移动,并且当球体碰到场景中的PickUp对象时将其隐藏。需要注意的是,该代码只包含了球体的移动和碰撞检测逻辑,还需要根据游戏需求添加其他逻辑和功能。
unity三维弹射小球游戏代码
以下是一个简单的Unity三维弹射小球游戏的代码示例:
```csharp
using UnityEngine;
using System.Collections;
public class BallController : MonoBehaviour {
public float speed; // 球体移动速度
public float jumpForce; // 球体弹跳力
private Rigidbody rb;
private bool isGrounded; // 是否在地面上
void Start () {
rb = GetComponent<Rigidbody>();
isGrounded = true; // 初始时球体在地面上
}
void FixedUpdate () {
// 获取用户输入
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
// 根据用户输入移动球体
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
rb.AddForce(movement * speed);
// 球体跳跃
if (Input.GetKeyDown(KeyCode.Space) && isGrounded) {
rb.AddForce(Vector3.up * jumpForce, ForceMode.Impulse);
isGrounded = false;
}
}
void OnCollisionEnter(Collision other) {
// 判断球体是否在地面上
if (other.gameObject.CompareTag("Ground")) {
isGrounded = true;
}
}
}
```
这段代码实现了用户控制球体移动,并且可以在空中进行一次弹跳。需要注意的是,该代码只包含了球体的移动和弹跳逻辑,还需要根据游戏需求添加其他逻辑和功能。此外,该代码还使用了碰撞检测来判断球体是否在地面上,需要在场景中添加一个Ground对象,并为其设置标签为"Ground"。
阅读全文
相关推荐







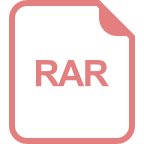
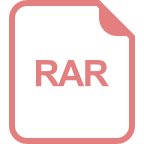
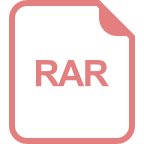






