html+js贪吃蛇示例代码
时间: 2023-07-27 09:07:44 浏览: 94
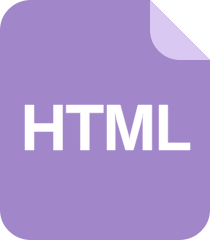
贪吃蛇html代码
以下是一个基于 HTML 和 JavaScript 的贪吃蛇示例代码:
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>贪吃蛇</title>
<style>
#canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript:
```javascript
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
const tileSize = 10;
const width = canvas.width / tileSize;
const height = canvas.height / tileSize;
let snake = [{ x: 5, y: 5 }];
let apple = { x: 10, y: 10 };
let direction = "right";
let score = 0;
function drawTile(x, y, color) {
ctx.fillStyle = color;
ctx.fillRect(x * tileSize, y * tileSize, tileSize, tileSize);
}
function drawSnake() {
snake.forEach(function (tile) {
drawTile(tile.x, tile.y, "green");
});
}
function drawApple() {
drawTile(apple.x, apple.y, "red");
}
function update() {
let snakeHead = { x: snake[0].x, y: snake[0].y };
switch (direction) {
case "up":
snakeHead.y--;
break;
case "down":
snakeHead.y++;
break;
case "left":
snakeHead.x--;
break;
case "right":
snakeHead.x++;
break;
}
// Check if snake hits wall
if (snakeHead.x < 0 || snakeHead.x >= width || snakeHead.y < 0 || snakeHead.y >= height) {
alert("Game Over!");
location.reload();
}
// Check if snake hits itself
for (let i = 1; i < snake.length; i++) {
if (snakeHead.x === snake[i].x && snakeHead.y === snake[i].y) {
alert("Game Over!");
location.reload();
}
}
snake.unshift(snakeHead);
// Check if snake eats apple
if (snakeHead.x === apple.x && snakeHead.y === apple.y) {
score++;
document.getElementById("score").innerHTML = "Score: " + score;
apple.x = Math.floor(Math.random() * width);
apple.y = Math.floor(Math.random() * height);
} else {
snake.pop();
}
}
function gameLoop() {
update();
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawSnake();
drawApple();
}
setInterval(gameLoop, 100);
document.addEventListener("keydown", function (event) {
switch (event.key) {
case "ArrowUp":
if (direction !== "down") {
direction = "up";
}
break;
case "ArrowDown":
if (direction !== "up") {
direction = "down";
}
break;
case "ArrowLeft":
if (direction !== "right") {
direction = "left";
}
break;
case "ArrowRight":
if (direction !== "left") {
direction = "right";
}
break;
}
});
```
这个示例代码实现了一个基本的贪吃蛇游戏,包括贪吃蛇的移动、撞墙判定、撞自身判定、吃苹果、计分等功能。你可以将代码保存为 `snake.html` 和 `snake.js`,在浏览器中打开 `snake.html` 运行游戏。
阅读全文
相关推荐
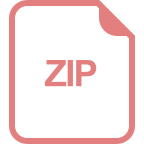
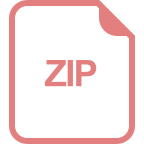
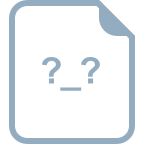
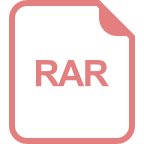
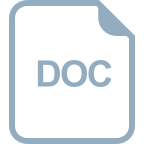
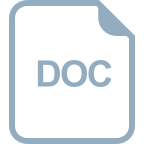
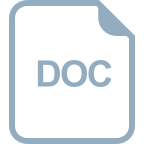

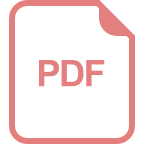
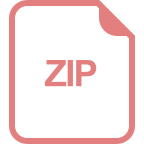
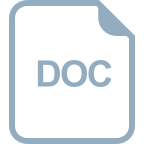
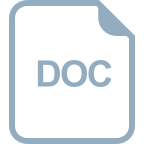
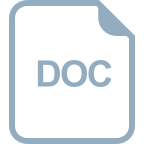
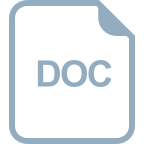
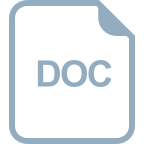
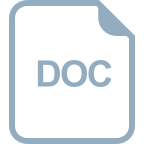