某班30个学生的学号为20220301-20220330,全部选修了Java程序设计课程,给出所有同学的成绩(可用随机数产生,范围60-100),请编写程序将本班各位同学的成绩按照从低到高排序打印输出。 要求:分别用List、Map、Set来实现,打印的信息包括学号、姓名和成绩。
时间: 2024-02-27 21:57:14 浏览: 23
List实现:
```
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
for (int i = 1; i <= 30; i++) {
Student student = new Student("202203" + String.format("%02d", i), "学生" + i, (int) (Math.random() * 41) + 60);
studentList.add(student);
}
Collections.sort(studentList);
for (Student student : studentList) {
System.out.println(student);
}
}
}
class Student implements Comparable<Student> {
private String studentNo;
private String name;
private int score;
public Student(String studentNo, String name, int score) {
this.studentNo = studentNo;
this.name = name;
this.score = score;
}
@Override
public int compareTo(Student o) {
return score - o.score;
}
@Override
public String toString() {
return "学号:" + studentNo + ",姓名:" + name + ",成绩:" + score;
}
}
```
Map实现:
```
import java.util.*;
public class Main {
public static void main(String[] args) {
Map<String, Student> studentMap = new TreeMap<>();
for (int i = 1; i <= 30; i++) {
Student student = new Student("202203" + String.format("%02d", i), "学生" + i, (int) (Math.random() * 41) + 60);
studentMap.put(student.getStudentNo(), student);
}
List<Student> studentList = new ArrayList<>(studentMap.values());
Collections.sort(studentList);
for (Student student : studentList) {
System.out.println(student);
}
}
}
class Student implements Comparable<Student> {
private String studentNo;
private String name;
private int score;
public Student(String studentNo, String name, int score) {
this.studentNo = studentNo;
this.name = name;
this.score = score;
}
public String getStudentNo() {
return studentNo;
}
@Override
public int compareTo(Student o) {
return score - o.score;
}
@Override
public String toString() {
return "学号:" + studentNo + ",姓名:" + name + ",成绩:" + score;
}
}
```
Set实现:
```
import java.util.*;
public class Main {
public static void main(String[] args) {
Set<Student> studentSet = new TreeSet<>();
for (int i = 1; i <= 30; i++) {
Student student = new Student("202203" + String.format("%02d", i), "学生" + i, (int) (Math.random() * 41) + 60);
studentSet.add(student);
}
for (Student student : studentSet) {
System.out.println(student);
}
}
}
class Student implements Comparable<Student> {
private String studentNo;
private String name;
private int score;
public Student(String studentNo, String name, int score) {
this.studentNo = studentNo;
this.name = name;
this.score = score;
}
@Override
public int compareTo(Student o) {
return score - o.score;
}
@Override
public String toString() {
return "学号:" + studentNo + ",姓名:" + name + ",成绩:" + score;
}
}
```
相关推荐
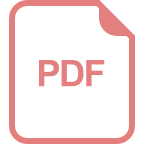
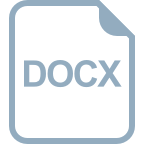
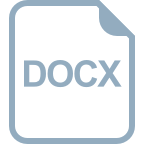














