getchar()输入abcde,输出是什么
时间: 2023-11-22 09:52:27 浏览: 39
如果使用C语言编写一个程序,其中包含一个 `getchar()` 函数,输入 `abcde`,则输出应该是 `abcde`。
这是因为 `getchar()` 函数会逐个读取输入流中的字符,并将其作为整数返回。在输入 `abcde` 时,`getchar()` 函数会依次读取字符 `a`、`b`、`c`、`d`、`e`,然后程序会将这些字符输出到屏幕上。
相关问题
用getchar和putchar输入输出
可以使用 `getchar()` 函数从标准输入读取一个字符,并使用 `putchar()` 函数将字符输出到标准输出。以下是一个示例代码:
```c
#include <stdio.h>
int main() {
int c;
printf("请输入一个字符:");
c = getchar();
printf("你输入的字符是:");
putchar(c);
return 0;
}
```
段代码首先用 `getchar()` 函数从标准输入读取一个字符,并将其存储在变量 `c` 中。然后使用 `putchar()` 函数将字符 `c` 输出到标准输出。注意,`getchar()` 和 `putchar()` 函数都是按字符方式操作的,它们返回和接受的都是 int 类型的值,因为它们可以表示额外的特殊值(例如EOF)以及字符值。
若getchar输入进数组的是345,putchar输出的是什么
如果输入进数组的是345,那么数组中存储的是字符'3'、字符'4'和字符'5',它们的ASCII码分别为51、52和53。如果依次使用putchar输出这三个字符,会输出345,即与输入的字符串相同。可以使用下面的代码实现:
```c
#include <stdio.h>
int main() {
char arr[3];
arr[0] = '3';
arr[1] = '4';
arr[2] = '5';
for (int i = 0; i < 3; i++) {
putchar(arr[i]);
}
return 0;
}
```
输出结果为:
```
345
```
相关推荐
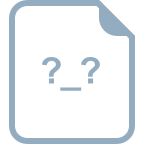
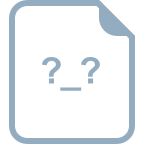
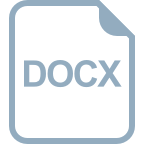












