def build_tile_cache(self): self.tiles = [] for files in tqdm(self.index, desc='Building/Checking Tile Cache'): file = files[0] cache_dir = self.cache_dir / file.stem lockfile = cache_dir / 'lock.file' if not cache_dir.is_dir() or lockfile.exists(): if lockfile.exists(): shutil.rmtree(cache_dir) cache_dir.mkdir(exist_ok=False) lockfile.touch()是什么意思
时间: 2024-02-14 20:27:00 浏览: 43
`cache_dir` 是一个目录,`lockfile` 是在 `cache_dir` 目录下的一个文件,用于标记该目录是否被其他进程占用。下面是对代码的解释:
- `if not cache_dir.is_dir() or lockfile.exists():` 检查 `cache_dir` 是否存在,如果不存在或者 `lockfile` 存在,则执行以下操作。
- `shutil.rmtree(cache_dir)` 删除 `cache_dir` 目录及其所有内容。
- `cache_dir.mkdir(exist_ok=False)` 创建一个新的 `cache_dir` 目录,如果该目录已存在则会引发异常。
- `lockfile.touch()` 创建一个空的 `lockfile` 文件。
这段代码的作用是检查并构建/检查缓存目录和锁文件,确保每个缓存目录是空的并且没有其他进程正在使用。
相关问题
class Tile(pygame.sprite.Sprite): def __init__(self,pos,groups,sprite_type,surface = pygame.Surface((TILESIZE,TILESIZE))): super().__init__(groups) self.sprite_type = sprite_type y_offset = HITBOX_OFFSET[sprite_type] self.image = surface if sprite_type == 'object': self.rect = self.image.get_rect(topleft = (pos[0],pos[1] - TILESIZE)) else: self.rect = self.image.get_rect(topleft = pos) self.hitbox = self.rect.inflate(0,y_offset)对该代码进行注释
这段代码是用 Python 编写的,定义了一个名为 Tile 的类。这个类用于在 Pygame 中显示游戏中的图像。
注释如下:
```python
class Tile(pygame.sprite.Sprite):
# 定义 Tile 类,继承 Pygame.sprite.Sprite 类
def __init__(self,pos,groups,sprite_type,surface = pygame.Surface((TILESIZE,TILESIZE))):
# Tile 类的构造方法,传入 pos、groups、sprite_type 和 surface 四个参数
# pos 表示 Tile 对象在游戏中的位置
# groups 表示 Tile 对象所属的组
# sprite_type 表示 Tile 对象的类型
# surface 表示 Tile 对象的表面,默认为大小为 TILESIZE 的 Surface 对象
super().__init__(groups) # 调用父类的构造方法,进行初始化
self.sprite_type = sprite_type # 设置 Tile 对象的 sprite_type 属性
y_offset = HITBOX_OFFSET[sprite_type] # 设置 Tile 对象的 y_offset 属性,值为 HITBOX_OFFSET[sprite_type]
self.image = surface # 设置 Tile 对象的 image 属性,值为传入的 surface 参数
if sprite_type == 'object':
# 若 sprite_type 为 'object',则将 Tile 对象的 rect 设置为在 pos 坐标左上角位置的矩形
# 同时将 Tile 对象的 hitbox 属性上下扩展了 HITBOX_OFFSET[sprite_type] 个像素
self.rect = self.image.get_rect(topleft = (pos[0],pos[1] - TILESIZE))
else:
# 否则,将 Tile 对象的 rect 直接设置为 pos 坐标的矩形
# 同时将 Tile 对象的 hitbox 属性上下扩展了 0 个像素
self.rect = self.image.get_rect(topleft = pos)
self.hitbox = self.rect.inflate(0,y_offset) # 设置 Tile 对象的 hitbox 属性,值为 rect 属性上下扩展了 y_offset 个像素后的矩形
```
该代码主要是设置 Tile 对象的属性,包括 sprite_type、image、rect 和 hitbox 等属性。其中,sprite_type 表示 Tile 对象的类型,image 表示 Tile 对象的表面,rect 表示 Tile 对象在游戏中的位置和大小,hitbox 表示 Tile 对象的碰撞检测区域。
class YSortCameraGroup(pygame.sprite.Group): def __init__(self): super().__init__() self.display_surface = pygame.display.get_surface() self.half_width = self.display_surface.get_size()[0] // 2 self.half_height = self.display_surface.get_size()[1] // 2 self.offset = pygame.math.Vector2() self.floor_surf = pygame.image.load('../graphics/tilemap/ground.png').convert() self.floor_rect = self.floor_surf.get_rect(topleft = (0,0))
这段代码定义了一个自定义的精灵组类 `YSortCameraGroup`,用于实现按照 Y 坐标排序的相机组。下面是对代码的详细解释:
```python
class YSortCameraGroup(pygame.sprite.Group):
def __init__(self):
super().__init__()
# 获取当前显示窗口
self.display_surface = pygame.display.get_surface()
# 计算窗口宽度和高度的一半
self.half_width = self.display_surface.get_size()[0] // 2
self.half_height = self.display_surface.get_size()[1] // 2
# 初始化偏移量
self.offset = pygame.math.Vector2()
# 加载地面贴图
self.floor_surf = pygame.image.load('../graphics/tilemap/ground.png').convert()
self.floor_rect = self.floor_surf.get_rect(topleft=(0, 0))
```
该类继承自 `pygame.sprite.Group` 类,因此可以进行精灵的分组管理。在类的初始化方法中,首先调用父类的 `__init__()` 方法进行初始化。然后,获取当前显示窗口并计算窗口宽度和高度的一半,以便后续计算精灵在屏幕上的位置。接着,初始化偏移量为一个 `pygame.math.Vector2()` 对象,用于控制相机的移动。最后,加载地面贴图并获取其矩形对象。
该类的作用是实现按照精灵在 Y 轴上的位置进行排序,并将其绘制到屏幕上。由于精灵在屏幕上的位置是相对于相机位置而言的,因此需要使用偏移量对其进行计算。
相关推荐











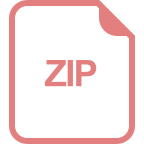
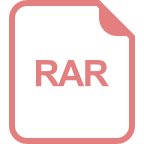