import numpy as np from sklearn.cluster import KMeans def compress_image(image, n_clusters): # 转换为一维向量 data = image.reshape(-1, 3) # 用 KMeans 算法将像素点聚类到 n_clusters 个簇中 kmeans = KMeans(n_clusters=5, random_state=0).fit(data) # 将每个像素点替换为所属簇的中心像素值 compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_]) # 将压缩后的一维向量转换回原图像的形状 compressed_image = compressed_data.reshape(image.shape) return compressed_imageimport matplotlib.pyplot as plt from PIL import Image # 读取图像 image = np.array(Image.open('0.jpg')) # 将图像数据归一化到 [0, 1] 范围内 image = image.astype('float32') / 255.0 # 压缩图像 compressed_image = compress_image(image_norm, n_clusters=16) # 显示压缩前后的图像 fig, ax = plt.subplots(1, 2, figsize=(10, 5)) ax[0].imshow(image) ax[0].set_title('Original Image') ax[1].imshow(compressed_image) ax[1].set_title('Compressed Image') plt.show()加上计算压缩率的功能
时间: 2024-02-17 19:00:53 浏览: 90
可以在 compress_image 函数中添加计算压缩率的功能,具体实现如下:
```
import numpy as np
from sklearn.cluster import KMeans
def compress_image(image, n_clusters):
# 转换为一维向量
data = image.reshape(-1, 3)
# 用 KMeans 算法将像素点聚类到 n_clusters 个簇中
kmeans = KMeans(n_clusters=n_clusters, random_state=0).fit(data)
# 将每个像素点替换为所属簇的中心像素值
compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_])
# 将压缩后的一维向量转换回原图像的形状
compressed_image = compressed_data.reshape(image.shape)
# 计算压缩率
compressed_size = compressed_data.nbytes
original_size = image.nbytes
compression_ratio = compressed_size / original_size
return compressed_image, compression_ratio
import matplotlib.pyplot as plt
from PIL import Image
# 读取图像
image = np.array(Image.open('0.jpg'))
# 将图像数据归一化到 [0, 1] 范围内
image = image.astype('float32') / 255.0
# 压缩图像
n_clusters = 5
compressed_image, compression_ratio = compress_image(image, n_clusters)
# 显示压缩前后的图像
fig, ax = plt.subplots(1, 2, figsize=(10, 5))
ax[0].imshow(image)
ax[0].set_title('Original Image')
ax[1].imshow(compressed_image)
ax[1].set_title('Compressed Image')
# 显示压缩率
plt.figtext(0.5, 0.9, f'Compression Ratio: {compression_ratio:.2%}', ha='center', fontsize=12)
plt.show()
```
在上述代码中,计算压缩率的代码为:
```
compressed_size = compressed_data.nbytes
original_size = image.nbytes
compression_ratio = compressed_size / original_size
```
其中,`compressed_data.nbytes` 表示压缩后的图像数据的字节数,`image.nbytes` 表示原始图像数据的字节数,压缩率即为两者之比。
阅读全文
相关推荐
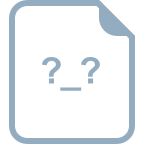
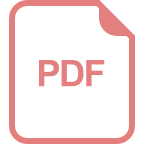
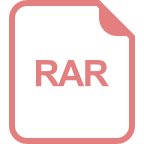
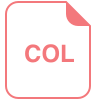
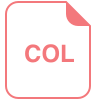
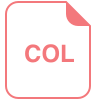
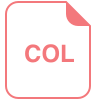
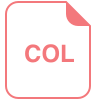
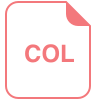
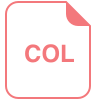

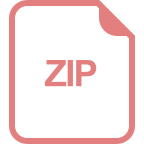
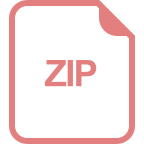
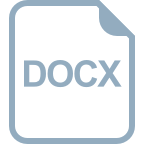
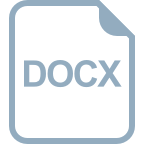