将一个形状为(417,556,3)的彩色图像,在jupyter notebooks上基于向量量化的压缩算法代码
时间: 2024-02-16 08:59:46 浏览: 68
以下是基于向量量化的图像压缩算法代码示例:
```python
import numpy as np
from sklearn.cluster import KMeans
def compress_image(image, n_clusters):
# 转换为一维向量
data = image.reshape(-1, 3)
# 用 KMeans 算法将像素点聚类到 n_clusters 个簇中
kmeans = KMeans(n_clusters=n_clusters, random_state=0).fit(data)
# 将每个像素点替换为所属簇的中心像素值
compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_])
# 将压缩后的一维向量转换回原图像的形状
compressed_image = compressed_data.reshape(image.shape)
return compressed_image
```
在上面的代码中,我们首先将彩色图像转换为一维向量,然后使用 KMeans 算法将像素点聚类到指定数量的簇中。每个像素点被替换为所属簇的中心像素值,从而实现压缩。最后,我们将压缩后的一维向量转换回原始图像的形状。
以下是使用示例:
```python
import matplotlib.pyplot as plt
from PIL import Image
# 读取图像
image = np.array(Image.open('image.jpg'))
# 压缩图像
compressed_image = compress_image(image, n_clusters=16)
# 显示压缩前后的图像
fig, ax = plt.subplots(1, 2, figsize=(10, 5))
ax[0].imshow(image)
ax[0].set_title('Original Image')
ax[1].imshow(compressed_image)
ax[1].set_title('Compressed Image')
plt.show()
```
在上面的代码中,我们使用了 matplotlib 和 PIL 库来读取和显示图像。我们将原始图像和压缩后的图像一起显示,以便比较它们的差异。
阅读全文
相关推荐
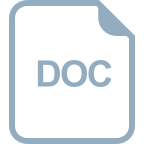
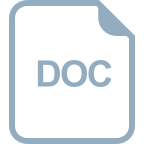
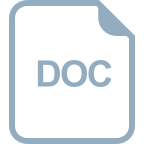















