import tkinter as tk root = tk.Tk() canvas = tk.Canvas(root, width=200, height=200) canvas.pack() rect = canvas.create_rectangle(50, 50, 150, 150, fill="blue") button = tk.Button(root, text="停止动画", command=root.quit) button.pack() dx = 5 dy = 5 while True: canvas.move(rect, dx, dy) canvas.update() # 如果矩形碰到了边界,就反弹回来 if canvas.coords(rect)[0] <= 0 or canvas.coords(rect)[2] >= 200: dx = -dx if canvas.coords(rect)[1] <= 0 or canvas.coords(rect)[3] >= 200: dy = -dy # 等待一段时间,让动画看起来更流畅 root.after(10) root.mainloop()
时间: 2023-05-22 10:02:15 浏览: 67
这段代码使用了Python的GUI库tkinter,创建了一个窗口和一个画布,并在画布上绘制了一个蓝色的矩形。同时还创建了一个按钮,点击该按钮可以停止动画。在while循环中,矩形会以每次移动5个像素的速度向右下方移动,直到程序被手动停止。
相关问题
tkinter root=tk.Tk() 怎么设置root的背景图片
要设置`tkinter`窗口的背景图片,可以使用`PIL`库中的`ImageTk`模块,将图片转换为`Tkinter`的PhotoImage对象,然后使用`Canvas`小部件将其放置在窗口上。
以下是一个简单的示例代码,用于将背景图片设置为`root`窗口的背景:
```python
from tkinter import *
from PIL import ImageTk, Image
root = Tk()
# 打开图片文件
img = Image.open("background.png")
# 将图片文件转换为PhotoImage对象
background_image = ImageTk.PhotoImage(img)
# 创建Canvas小部件
canvas = Canvas(root, width=img.size[0], height=img.size[1])
canvas.pack()
# 在Canvas上放置背景图片
canvas.create_image(0, 0, anchor=NW, image=background_image)
# 设置窗口大小为背景图片大小
root.geometry("{}x{}".format(img.size[0], img.size[1]))
# 显示窗口
root.mainloop()
```
在上面的示例代码中,我们首先打开背景图片文件,然后使用`ImageTk`模块将其转换为`PhotoImage`对象。接下来,我们创建一个`Canvas`小部件,并将其大小设置为背景图片的大小。最后,我们在`Canvas`上放置背景图片,并设置窗口大小为背景图片大小。运行代码后,就可以看到窗口的背景图片已经被设置了。
import tkinter as tk root = tk.Tk() # 创建一个画布和一个滚动条 canvas = tk.Canvas(root, width=400, height=300) scrollbar = tk.Scrollbar(root, orient='vertical', command=canvas.yview) canvas.config(yscrollcommand=scrollbar.set) scrollbar.pack(side='right', fill='y') canvas.pack(side='left', fill='both', expand=True) # 在画布中添加一个文本框 text = tk.Text(canvas) text.pack(side='left', fill='both', expand=True) canvas.create_window((0, 0), window=text, anchor='nw') # 添加一些文本到文本框中 for i in range(50): text.insert('end', f'Line {i}\n') # 配置文本框的滚动区域 text.config(yscrollcommand=scrollbar.set) text.config(height=10) text.config(width=30) root.mainloop()为什么滚轮不能鼠标滑动
这是因为你需要将滚轮绑定到 canvas 上才能使其滚动。可以使用以下代码将滚轮绑定到 canvas 上:
```
canvas.bind_all("<MouseWheel>", lambda event: canvas.yview_scroll(int(-1*(event.delta/120)), "units"))
```
将上述代码添加到你的程序中,就可以使用鼠标滚轮来滚动 canvas 了。
相关推荐
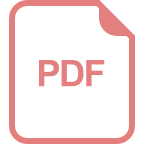
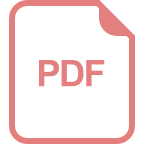
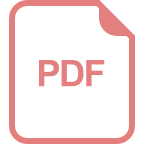













