遗传算法轮盘tsp问题
时间: 2023-12-22 10:04:23 浏览: 37
遗传算法是一种基于自然选择和遗传学原理的优化算法,可以用于解决TSP问题。其中轮盘赌选择是遗传算法中的一种选择策略,可以用于选择优秀的个体进行交叉和变异。
以下是遗传算法解决TSP问题的步骤:
1.初始化种群:随机生成一定数量的路径作为初始种群。
2.计算适应度:对于每个路径,计算其总长度作为适应度。
3.选择操作:使用轮盘赌选择策略,选择适应度较高的个体进行交叉和变异。
4.交叉操作:随机选择两个个体进行交叉,生成新的个体。
5.变异操作:对于某些个体,随机选择其中的一个节点进行变异,生成新的个体。
6.重复步骤2-5,直到达到预设的迭代次数或找到最优解。
以下是Python代码示例:
```python
import random
# TSP问题的距离矩阵
distance_matrix = [[0, 2, 9, 10],
[1, 0, 6, 4],
[15, 7, 0, 8],
[6, 3, 12, 0]]
# 遗传算法参数
population_size = 10 # 种群大小
elite_size = 2 # 精英个体数量
mutation_rate = 0.1 # 变异率
generations = 100 # 迭代次数
# 初始化种群
def initial_population(population_size, distance_matrix):
population = []
for i in range(population_size):
path = list(range(len(distance_matrix)))
random.shuffle(path)
population.append(path)
return population
# 计算路径长度
def path_length(path, distance_matrix):
length = 0
for i in range(len(path)-1):
length += distance_matrix[path[i]][path[i+1]]
length += distance_matrix[path[-1]][path[0]]
return length
# 计算适应度
def fitness(population, distance_matrix):
fitness_scores = []
for path in population:
fitness_scores.append(1/path_length(path, distance_matrix))
return fitness_scores
# 轮盘赌选择
def selection(population, fitness_scores, elite_size):
elite = []
selection_probs = [score/sum(fitness_scores) for score in fitness_scores]
for i in range(elite_size):
elite_index = selection_probs.index(max(selection_probs))
elite.append(population[elite_index])
population.pop(elite_index)
fitness_scores.pop(elite_index)
selection_probs.pop(elite_index)
parents = []
for i in range(len(population)):
parent1 = random.choices(population, weights=selection_probs)[0]
parent2 = random.choices(population, weights=selection_probs)[0]
parents.append((parent1, parent2))
return elite, parents
# 交叉操作
def crossover(parents):
children = []
for parent1, parent2 in parents:
child = [-1] * len(parent1)
start = random.randint(0, len(parent1)-1)
end = random.randint(0, len(parent1)-1)
if start > end:
start, end = end, start
for i in range(start, end+1):
child[i] = parent1[i]
for i in range(len(parent2)):
if parent2[i] not in child:
for j in range(len(child)):
if child[j] == -1:
child[j] = parent2[i]
break
children.append(child)
return children
# 变异操作
def mutation(children, mutation_rate):
for i in range(len(children)):
if random.random() < mutation_rate:
index1 = random.randint(0, len(children[i])-1)
index2 = random.randint(0, len(children[i])-1)
children[i][index1], children[i][index2] = children[i][index2], children[i][index1]
return children
# 遗传算法主函数
def genetic_algorithm(distance_matrix, population_size, elite_size, mutation_rate, generations):
population = initial_population(population_size, distance_matrix)
for i in range(generations):
fitness_scores = fitness(population, distance_matrix)
elite, parents = selection(population, fitness_scores, elite_size)
children = crossover(parents)
children = mutation(children, mutation_rate)
population = elite + children
fitness_scores = fitness(population, distance_matrix)
best_index = fitness_scores.index(max(fitness_scores))
best_path = population[best_index]
return best_path, path_length(best_path, distance_matrix)
# 运行遗传算法
best_path, best_length = genetic_algorithm(distance_matrix, population_size, elite_size, mutation_rate, generations)
print("Best path:", best_path)
print("Best length:", best_length)
```
相关推荐
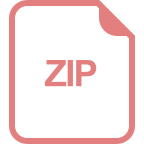
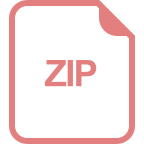













