springboot实现图片上传接口
时间: 2023-10-14 22:31:14 浏览: 102
在Spring Boot中实现图片上传功能,可以使用`MultipartFile`接口来处理上传的文件。以下是一个简单的实现方式:
1. 在`pom.xml`文件中添加`spring-boot-starter-web`和`spring-boot-starter-tomcat`依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
```
2. 创建一个Controller类,实现图片上传接口:
```java
@RestController
public class ImageController {
@PostMapping("/upload")
public String uploadImage(@RequestParam("file") MultipartFile file) throws IOException {
// 获取上传文件的原始文件名
String originalFilename = file.getOriginalFilename();
// 获取上传文件的扩展名
String extension = FilenameUtils.getExtension(originalFilename);
// 生成新的文件名
String newFilename = UUID.randomUUID().toString() + "." + extension;
// 保存文件到本地磁盘
File newFile = new File("C:/uploads/" + newFilename);
file.transferTo(newFile);
// 返回上传成功的消息
return "上传成功";
}
}
```
3. 在`application.properties`文件中配置上传文件的最大大小和临时存储路径:
```
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
spring.servlet.multipart.location=C:/uploads/tmp
```
以上代码中,我们使用了`@RequestParam("file")`注解来接收上传的文件,然后利用`MultipartFile`接口提供的方法获取文件名、扩展名等信息,并将文件保存到本地磁盘中。最后返回一个上传成功的消息。
需要注意的是,上传文件的最大大小和临时存储路径需要在`application.properties`文件中进行配置。在本例中,我们将上传文件的最大大小限制为10MB,临时存储路径为`C:/uploads/tmp`。
阅读全文
相关推荐
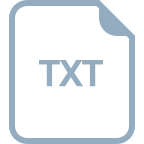
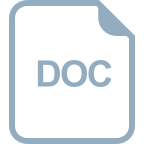

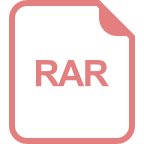


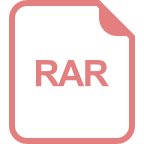
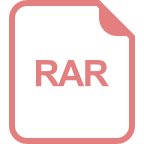
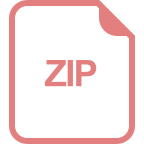
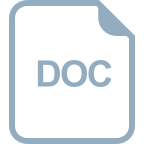
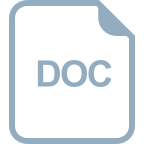
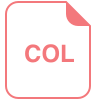



