那么帮我在C90标准下写一个可以实现加减乘除并且判断括号优先级的程序,要求使用顺序栈,帮我详细注释每一步
时间: 2023-06-09 15:07:16 浏览: 126
好的,请问你需要的是C语言的吗?还是其他编程语言?
如果是C语言的话,下面是一个可以实现加减乘除并且判断括号优先级的程序,使用顺序栈实现。
```c
#include <stdio.h>
#include <ctype.h>
#define STACK_SIZE 100
int stack[STACK_SIZE]; // 定义一个顺序栈
int top = -1; // 栈顶指针初始化为-1
void push(int value) // 入栈操作
{
if (top >= STACK_SIZE - 1) { // 判断栈是否已满
printf("Stack overflow\n");
return;
}
stack[++top] = value;
}
int pop() // 出栈操作
{
if (top == -1) { // 判断栈是否为空
printf("Stack underflow\n");
return -1;
}
return stack[top--];
}
int getPriority(char operator) // 获取运算符优先级的函数
{
switch (operator) {
case '(': return 0;
case '+':
case '-': return 1;
case '*':
case '/': return 2;
default: return -1;
}
}
int main()
{
char expression[STACK_SIZE]; // 定义一个字符数组用于存储表达式
int i, len, operand1, operand2, result;
char operator;
printf("请输入一个包含加减乘除以及括号的表达式:");
scanf("%s", expression); // 读取表达式
len = strlen(expression);
for (i = 0; i < len; i++) {
if (isdigit(expression[i])) { // 如果是数字,将其入栈
push(expression[i] - '0');
}
else if (expression[i] == '(') { // 如果是左括号,将其入栈
push(expression[i]);
}
else if (expression[i] == '+' || expression[i] == '-' || expression[i] == '*' || expression[i] == '/') { // 如果是运算符
while (top != -1 && stack[top] != '(' && getPriority(stack[top]) >= getPriority(expression[i])) { // 如果栈非空且栈顶元素不是左括号且栈顶运算符优先级不小于当前运算符优先级
operand2 = pop(); // 出栈两个操作数
operand1 = pop();
switch (stack[top + 1]) { // 根据运算符进行计算
case '+': result = operand1 + operand2; break;
case '-': result = operand1 - operand2; break;
case '*': result = operand1 * operand2; break;
case '/': result = operand1 / operand2; break;
}
push(result); // 将计算结果入栈
}
push(expression[i]); // 计算完之后再把当前运算符入栈
}
else if (expression[i] == ')') { // 如果是右括号
while (stack[top] != '(') { // 直到遇到左括号
operand2 = pop(); // 出栈两个操作数
operand1 = pop();
switch (stack[top + 1]) { // 根据运算符进行计算
case '+': result = operand1 + operand2; break;
case '-': result = operand1 - operand2; break;
case '*': result = operand1 * operand2; break;
case '/': result = operand1 / operand2; break;
}
push(result); // 将计算结果入栈
}
pop(); // 出栈左括号
}
}
while (top != -1) { // 最终计算结果
operand2 = pop(); // 出栈两个操作数
operand1 = pop();
switch (stack[top + 1]) { // 根据运算符进行计算
case '+': result = operand1 + operand2; break;
case '-': result = operand1 - operand2; break;
case '*': result = operand1 * operand2; break;
case '/': result = operand1 / operand2; break;
}
push(result); // 将计算结果入栈
}
printf("计算结果为:%d\n", pop()); // 输出最终结果
return 0;
}
```
这个程序使用了一个顺序栈来存储操作数和运算符,并且根据括号优先级来进行计算。详细注释已经添加到代码中,供你参考。
阅读全文
相关推荐


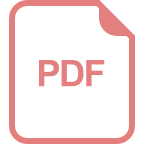
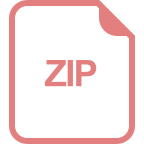
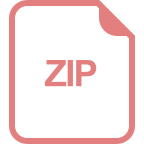
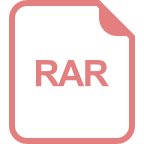
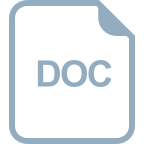
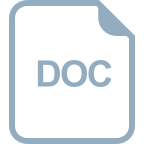
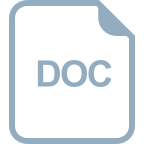






