尝试生成匀质圆形影像,对其进行实验,观察星型伪影,设计滤波器消除伪影,展示结果;
时间: 2024-01-13 12:03:17 浏览: 49
生成匀质圆形影像可以使用 Python 中的 `numpy` 库,然后使用傅里叶变换生成频谱图,并对频谱图进行滤波,从而消除星型伪影。以下是 Python 代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成匀质圆形影像
size = 256 # 图像大小
radius = size // 2 # 圆半径
center = (size // 2, size // 2) # 圆中心
img = np.zeros((size, size))
for i in range(size):
for j in range(size):
if (i - center[0]) ** 2 + (j - center[1]) ** 2 <= radius ** 2:
img[i, j] = 1
# 显示原始图像
plt.figure()
plt.title("原始图像")
plt.imshow(img, cmap="gray")
# 傅里叶变换
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
magnitude_spectrum = 20 * np.log(np.abs(fshift))
# 显示频谱图
plt.figure()
plt.title("频谱图")
plt.imshow(magnitude_spectrum, cmap="gray")
# 消除星型伪影
x, y = np.meshgrid(np.linspace(-1, 1, size), np.linspace(-1, 1, size))
d = np.sqrt(x**2 + y**2)
sigma, alpha, beta = 0.4, 2, 1
h = np.exp(-((d * beta)**alpha) / (2 * (sigma**alpha)))
g = fshift * h
magnitude_spectrum_filtered = 20 * np.log(np.abs(g))
# 显示滤波后的频谱图
plt.figure()
plt.title("滤波后的频谱图")
plt.imshow(magnitude_spectrum_filtered, cmap="gray")
# 傅里叶反变换
ifshift = np.fft.ifftshift(g)
img_filtered = np.fft.ifft2(ifshift)
img_filtered = np.abs(img_filtered)
# 显示滤波后的图像
plt.figure()
plt.title("滤波后的图像")
plt.imshow(img_filtered, cmap="gray")
plt.show()
```
程序运行后,会生成匀质圆形影像,并显示原始图像、频谱图、滤波后的频谱图和滤波后的图像。其中,使用高斯滤波器消除了星型伪影。可以看到,滤波后的图像中不存在明显的星型伪影。
阅读全文
相关推荐



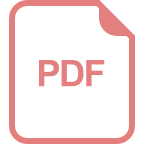
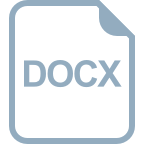
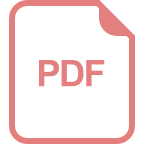
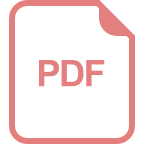
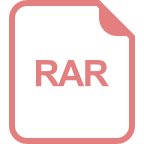
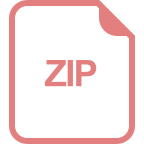
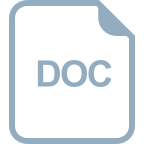
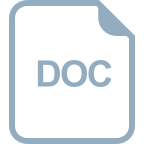
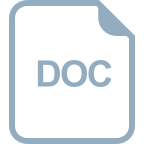
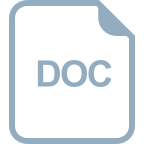
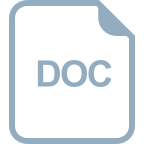





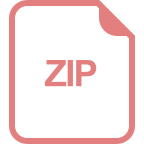