编写一个俄罗斯方块小游戏
时间: 2024-03-11 08:20:48 浏览: 59
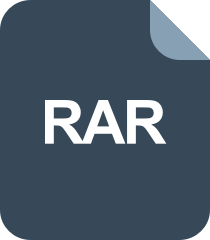
俄罗斯方块小游戏
好的,俄罗斯方块是一款经典的游戏,我们可以用 Python 和 Pygame 来编写一个简单的版本。
首先,我们需要安装 Pygame 库。可以在命令行中输入以下命令安装:
```
pip install pygame
```
接下来,我们来编写游戏逻辑:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 600
# 方块大小
BLOCK_SIZE = 20
# 方块种类和颜色
BLOCK_TYPES = [
[[1, 1, 1], [0, 1, 0]],
[[2, 2, 0], [0, 2, 2]],
[[0, 3, 3], [3, 3, 0]],
[[4, 4], [4, 4]],
[[5, 5, 5, 5]],
[[0, 6, 0], [6, 6, 6]],
[[7, 7], [7, 7]]
]
BLOCK_COLORS = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 游戏区域大小
GAME_AREA_WIDTH = BLOCK_SIZE * 10
GAME_AREA_HEIGHT = BLOCK_SIZE * 20
# 游戏区域左上角坐标
GAME_AREA_X = (WINDOW_WIDTH - GAME_AREA_WIDTH) // 2
GAME_AREA_Y = WINDOW_HEIGHT - GAME_AREA_HEIGHT - BLOCK_SIZE
# 创建游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 定义方块类
class Block:
def __init__(self, block_type, x, y):
self.block_type = block_type
self.color = BLOCK_COLORS[block_type]
self.rotation = 0
self.x = x
self.y = y
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.block_type)
def get_current_shape(self):
return self.block_type[self.rotation]
def move_left(self):
self.x -= BLOCK_SIZE
def move_right(self):
self.x += BLOCK_SIZE
def move_down(self):
self.y += BLOCK_SIZE
def draw(self):
shape = self.get_current_shape()
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
x = self.x + j * BLOCK_SIZE
y = self.y + i * BLOCK_SIZE
pygame.draw.rect(screen, self.color, (x, y, BLOCK_SIZE, BLOCK_SIZE))
# 定义游戏区域类
class GameArea:
def __init__(self):
self.blocks = [[0] * 10 for _ in range(20)]
def is_valid_position(self, block):
shape = block.get_current_shape()
x = block.x // BLOCK_SIZE
y = block.y // BLOCK_SIZE
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
if x + j < 0 or x + j >= 10 or y + i >= 20 or self.blocks[y + i][x + j] != 0:
return False
return True
def add_block(self, block):
shape = block.get_current_shape()
x = block.x // BLOCK_SIZE
y = block.y // BLOCK_SIZE
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
self.blocks[y + i][x + j] = block.block_type
def remove_complete_lines(self):
complete_lines = []
for i in range(len(self.blocks)):
if all(self.blocks[i]):
complete_lines.append(i)
for i in sorted(complete_lines, reverse=True):
del self.blocks[i]
self.blocks.insert(0, [0] * 10)
def draw(self):
for i in range(len(self.blocks)):
for j in range(len(self.blocks[i])):
if self.blocks[i][j] != 0:
x = GAME_AREA_X + j * BLOCK_SIZE
y = GAME_AREA_Y + i * BLOCK_SIZE
pygame.draw.rect(screen, BLOCK_COLORS[self.blocks[i][j]], (x, y, BLOCK_SIZE, BLOCK_SIZE))
# 初始化游戏区域和当前方块
game_area = GameArea()
current_block = Block(random.randint(0, len(BLOCK_TYPES) - 1), GAME_AREA_X + 4 * BLOCK_SIZE, GAME_AREA_Y)
# 游戏循环
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_block.move_left()
if not game_area.is_valid_position(current_block):
current_block.move_right()
elif event.key == pygame.K_RIGHT:
current_block.move_right()
if not game_area.is_valid_position(current_block):
current_block.move_left()
elif event.key == pygame.K_UP:
current_block.rotate()
if not game_area.is_valid_position(current_block):
current_block.rotate()
current_block.rotate()
current_block.rotate()
elif event.key == pygame.K_DOWN:
current_block.move_down()
if not game_area.is_valid_position(current_block):
current_block.move_up()
game_area.add_block(current_block)
game_area.remove_complete_lines()
current_block = Block(random.randint(0, len(BLOCK_TYPES) - 1), GAME_AREA_X + 4 * BLOCK_SIZE, GAME_AREA_Y)
# 绘制背景和游戏区域
screen.fill((255, 255, 255))
pygame.draw.rect(screen, (0, 0, 0), (GAME_AREA_X, GAME_AREA_Y, GAME_AREA_WIDTH, GAME_AREA_HEIGHT), 1)
game_area.draw()
# 绘制当前方块
current_block.move_down()
if not game_area.is_valid_position(current_block):
current_block.move_up()
game_area.add_block(current_block)
game_area.remove_complete_lines()
current_block = Block(random.randint(0, len(BLOCK_TYPES) - 1), GAME_AREA_X + 4 * BLOCK_SIZE, GAME_AREA_Y)
if not game_area.is_valid_position(current_block):
pygame.quit()
exit()
current_block.draw()
# 刷新屏幕
pygame.display.update()
# 控制帧率
clock.tick(10)
```
运行上述代码,就可以开始玩俄罗斯方块了。使用左右箭头键移动方块,上箭头键旋转方块,下箭头键加速下落。
阅读全文
相关推荐
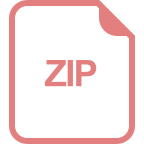



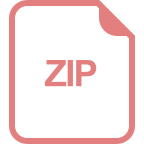
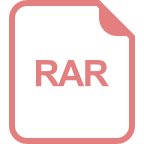






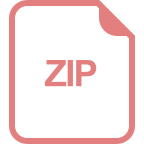
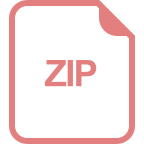
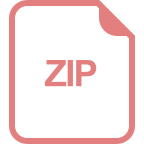
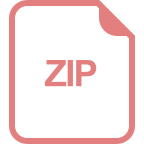
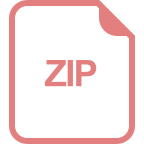
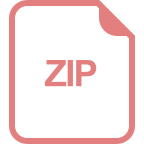