用Java写一个选座售票的代码
时间: 2024-05-04 18:16:13 浏览: 10
好的,我会尽力回答你的问题。以下是一个简单的用Java写的选座售票代码示例:
```
import java.util.Scanner;
public class SeatBookingSystem {
private static final int NUM_ROWS = 10;
private static final int SEATS_PER_ROW = 8;
private boolean[][] seats;
public SeatBookingSystem() {
seats = new boolean[NUM_ROWS][SEATS_PER_ROW];
for (int i = 0; i < NUM_ROWS; i++) {
for (int j = 0; j < SEATS_PER_ROW; j++) {
seats[i][j] = false;
}
}
}
public void printSeatingPlan() {
System.out.println("\nSeats:\n");
for (int i = 0; i < NUM_ROWS; i++) {
System.out.printf("%-3d", i + 1);
for (int j = 0; j < SEATS_PER_ROW; j++) {
if (seats[i][j]) {
System.out.print("[X]");
} else {
System.out.print("[ ]");
}
}
System.out.println();
}
}
public void bookSeat(int row, int seat) {
if (row < 1 || row > NUM_ROWS || seat < 1 || seat > SEATS_PER_ROW) {
System.out.println("Error: Invalid row or seat number.");
} else if (seats[row - 1][seat - 1]) {
System.out.println("Error: Seat is already booked.");
} else {
seats[row - 1][seat - 1] = true;
System.out.println("Seat booked.");
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
SeatBookingSystem system = new SeatBookingSystem();
int row, seat;
while (true) {
system.printSeatingPlan();
System.out.print("\nEnter row number (1-10) or 0 to exit: ");
row = scanner.nextInt();
if (row == 0) {
break;
}
System.out.print("Enter seat number (1-8): ");
seat = scanner.nextInt();
system.bookSeat(row, seat);
System.out.println("Press any key to continue...");
scanner.nextLine();
scanner.nextLine();
}
}
}
```
这个代码实现了一个简单的选座售票系统,可以打印出座位图,让用户输入座位号进行预订操作。座位状态保存在一个二维布尔数组中,true表示已经被预订,false表示还未被预订。用户输入row和seat后,调用bookSeat方法进行预订,如果座位已经被预订,则输出错误信息。预订成功后,更改座位状态为已预订,并输出成功信息。用户输入0退出程序。
相关推荐
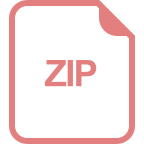
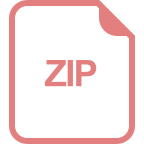














