给我一个Python和sql sever之间的一个学生信息管理系统
时间: 2024-03-12 15:49:16 浏览: 81
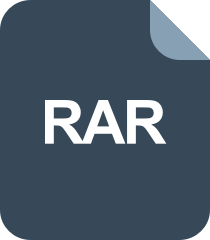
python+SQLsever+tkinter学生宿舍管理系统

好的,以下是一个简单的Python和SQL Server之间的学生信息管理系统的示例:
首先,我们需要创建一个数据库,以及一个名为“students”的表,其中包含学生的姓名和成绩:
```
CREATE DATABASE StudentDB
GO
USE StudentDB
GO
CREATE TABLE students (
id INT PRIMARY KEY IDENTITY,
name VARCHAR(50),
score INT
)
GO
```
接下来,我们可以使用Python连接到数据库,并创建一个简单的用户界面,允许用户添加,删除和查找学生信息:
```python
import pyodbc
# 连接到数据库
server = 'localhost'
database = 'StudentDB'
username = 'sa'
password = 'password'
cnxn = pyodbc.connect('DRIVER={SQL Server};SERVER='+server+';DATABASE='+database+';UID='+username+';PWD='+ password)
# 添加学生信息
def add_student(name, score):
cursor = cnxn.cursor()
cursor.execute("INSERT INTO students (name, score) VALUES (?, ?)", (name, score))
cnxn.commit()
# 删除学生信息
def delete_student(id):
cursor = cnxn.cursor()
cursor.execute("DELETE FROM students WHERE id=?", (id,))
cnxn.commit()
# 查找学生信息
def find_student(name):
cursor = cnxn.cursor()
cursor.execute("SELECT * FROM students WHERE name=?", (name,))
row = cursor.fetchone()
if row:
print("ID:", row[0], "Name:", row[1], "Score:", row[2])
else:
print("No student found with name", name)
# 用户界面
while True:
print("\nStudent Information Management System")
print("1. Add student")
print("2. Delete student")
print("3. Find student")
print("4. Exit")
choice = input("Enter your choice (1-4): ")
if choice == '1':
name = input("Enter student name: ")
score = int(input("Enter student score: "))
add_student(name, score)
print("Student added successfully!")
elif choice == '2':
id = int(input("Enter student ID: "))
delete_student(id)
print("Student deleted successfully!")
elif choice == '3':
name = input("Enter student name: ")
find_student(name)
elif choice == '4':
break
else:
print("Invalid choice. Please try again.")
```
这个示例只是一个基础的框架,你可以根据自己的需求对其进行扩展和修改。
阅读全文
相关推荐
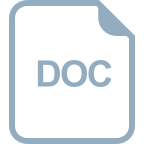
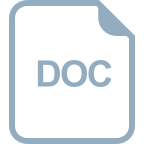
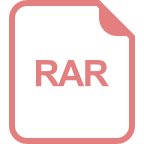
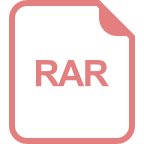
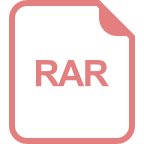












