使用Java手写一个spring的源码实现
时间: 2024-05-11 12:18:13 浏览: 16
要手写一个Spring的源码实现是一个非常复杂的任务,需要对Spring的核心原理和设计思想有深入的理解。以下是一个简单的Spring源码实现的示例,仅供参考。
首先,我们需要创建一个核心的容器类来管理Bean的生命周期和依赖注入。这个容器类需要实现BeanFactory接口,提供getBean()方法来获取Bean对象。
```java
public interface BeanFactory {
Object getBean(String name);
}
```
接下来,我们需要实现一个简单的Bean定义类,用于描述Bean的属性和依赖关系。
```java
public class BeanDefinition {
private Class<?> beanClass;
private Map<String, Object> properties = new HashMap<>();
private List<PropertyValue> propertyValues = new ArrayList<>();
public void setBeanClass(Class<?> beanClass) {
this.beanClass = beanClass;
}
public Class<?> getBeanClass() {
return beanClass;
}
public void addProperty(String name, Object value) {
properties.put(name, value);
}
public Object getProperty(String name) {
return properties.get(name);
}
public List<PropertyValue> getPropertyValues() {
return propertyValues;
}
public void addPropertyValue(PropertyValue propertyValue) {
propertyValues.add(propertyValue);
}
}
public class PropertyValue {
private final String name;
private final Object value;
public PropertyValue(String name, Object value) {
this.name = name;
this.value = value;
}
public String getName() {
return name;
}
public Object getValue() {
return value;
}
}
```
然后,我们需要实现一个简单的BeanFactory实现类,用于创建和管理Bean对象。这个实现类需要读取Bean定义信息,创建Bean实例,并将其保存在一个Map中。
```java
public class SimpleBeanFactory implements BeanFactory {
private final Map<String, BeanDefinition> beanDefinitions = new HashMap<>();
private final Map<String, Object> beans = new HashMap<>();
public SimpleBeanFactory(String configLocation) {
loadBeanDefinitions(configLocation);
createBeans();
}
private void loadBeanDefinitions(String configLocation) {
// 从配置文件中读取Bean定义信息
}
private void createBeans() {
for (String beanName : beanDefinitions.keySet()) {
createBean(beanName);
}
}
private Object createBean(String beanName) {
BeanDefinition beanDefinition = beanDefinitions.get(beanName);
Class<?> beanClass = beanDefinition.getBeanClass();
Object bean = createInstance(beanClass);
applyPropertyValues(bean, beanDefinition.getPropertyValues());
beans.put(beanName, bean);
return bean;
}
private Object createInstance(Class<?> beanClass) {
try {
return beanClass.newInstance();
} catch (InstantiationException | IllegalAccessException e) {
throw new RuntimeException(e);
}
}
private void applyPropertyValues(Object bean, List<PropertyValue> propertyValues) {
for (PropertyValue propertyValue : propertyValues) {
String propertyName = propertyValue.getName();
Object value = propertyValue.getValue();
setPropertyValue(bean, propertyName, value);
}
}
private void setPropertyValue(Object bean, String propertyName, Object value) {
try {
BeanUtils.setProperty(bean, propertyName, value);
} catch (IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException(e);
}
}
@Override
public Object getBean(String name) {
return beans.get(name);
}
}
```
最后,我们需要实现一个简单的测试类来验证我们的实现是否正确。
```java
public class SimpleBeanFactoryTest {
@Test
public void testGetBean() {
BeanFactory beanFactory = new SimpleBeanFactory("classpath:beans.xml");
TestBean testBean = (TestBean) beanFactory.getBean("testBean");
assertNotNull(testBean);
assertNotNull(testBean.getDependency());
}
}
```
这只是一个简单的Spring源码实现示例,实际上Spring的源码实现要复杂得多,涉及到很多高级特性和设计模式。
相关推荐
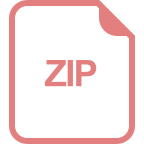
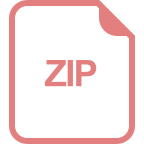
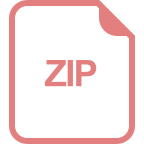
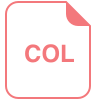
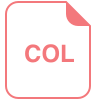
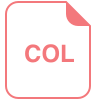











