自己使用Java手写实现简单正则表达式引擎
时间: 2023-12-09 22:07:03 浏览: 31
实现一个简单的正则表达式引擎,可以分为以下几个步骤:
1. 将正则表达式转换为后缀表达式
2. 使用后缀表达式匹配输入字符串
以下是一个简单的实现示例:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
public class SimpleRegexEngine {
private String regex;
private List<String> postfix;
public SimpleRegexEngine(String regex) {
this.regex = regex;
this.postfix = new ArrayList<>();
convertToPostfix();
}
private void convertToPostfix() {
Stack<Character> stack = new Stack<>();
for (int i = 0; i < regex.length(); i++) {
char c = regex.charAt(i);
if (isOperator(c)) {
while (!stack.isEmpty() && priority(stack.peek()) >= priority(c)) {
postfix.add(stack.pop().toString());
}
stack.push(c);
} else if (c == '(') {
stack.push(c);
} else if (c == ')') {
while (!stack.isEmpty() && stack.peek() != '(') {
postfix.add(stack.pop().toString());
}
stack.pop();
} else {
postfix.add(Character.toString(c));
}
}
while (!stack.isEmpty()) {
postfix.add(stack.pop().toString());
}
}
private boolean isOperator(char c) {
return c == '|' || c == '*' || c == '+';
}
private int priority(char c) {
switch (c) {
case '|':
return 1;
case '+':
case '*':
return 2;
default:
return 0;
}
}
public boolean match(String input) {
Stack<Boolean> stack = new Stack<>();
for (String token : postfix) {
char c = token.charAt(0);
if (isOperator(c)) {
boolean b1 = stack.pop();
boolean b2 = c == '|' ? stack.pop() : true;
stack.push(b1 || b2);
} else if (c == '+') {
stack.push(stack.pop() && matchChar(input, stack.size()));
} else if (c == '*') {
stack.push(true);
while (matchChar(input, stack.size())) {
stack.pop();
stack.push(true);
}
} else {
stack.push(matchChar(input, stack.size()));
}
}
return stack.pop() && input.length() == stack.size();
}
private boolean matchChar(String input, int index) {
if (index >= input.length()) {
return false;
}
char c = input.charAt(index);
return regex.charAt(index) == '.' || regex.charAt(index) == c;
}
public static void main(String[] args) {
SimpleRegexEngine regexEngine = new SimpleRegexEngine("(a|b)*abb");
System.out.println(regexEngine.match("aabb")); // true
System.out.println(regexEngine.match("ababb")); // true
System.out.println(regexEngine.match("abab")); // false
}
}
```
在上面的代码中,我们首先将输入的正则表达式转换为后缀表达式,然后使用栈来匹配输入字符串。在匹配过程中,我们使用了三种操作符:`|` 表示或,`*` 表示重复零次或多次,`+` 表示重复一次或多次。我们还处理了`.` 表示匹配任意字符的情况。
该实现并不完整,可能无法支持某些正则表达式语法。但是这可以作为一个简单的起点,以帮助您更好地理解正则表达式引擎的工作原理。
相关推荐
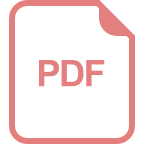
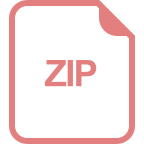
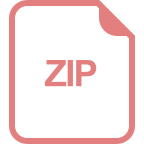














