创建一个学生结构体,如下所示。 struct stu{ int id; //学号 char name[20]; //姓名 int age; //年龄 int score; //成绩 }; 然后从键盘录入两名学生的信息,最后将上述信息格式化写入到文件。写入格式要求:将每个同学的信息写入到文件中的一行,每行中的各个数据之间以空格分隔。目前,已经完成了main函数的编写,请编程实现“stdinForStu”函数和“writeFileForStu”函数。
时间: 2023-11-22 15:55:41 浏览: 132
好的,这是stdinForStu和writeFileForStu的实现代码:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STU_NUM 2
struct stu{
int id; //学号
char name[20]; //姓名
int age; //年龄
int score; //成绩
};
void stdinForStu(struct stu *s)
{
printf("请输入学生信息:\n");
printf("学号:");
scanf("%d", &s->id);
printf("姓名:");
scanf("%s", s->name);
printf("年龄:");
scanf("%d", &s->age);
printf("成绩:");
scanf("%d", &s->score);
}
void writeFileForStu(struct stu *s, const char *filename)
{
FILE *fp;
fp = fopen(filename, "w");
if(fp == NULL) {
printf("无法打开文件!\n");
exit(1);
}
for(int i=0; i<MAX_STU_NUM; i++) {
fprintf(fp, "%d %s %d %d\n", s[i].id, s[i].name, s[i].age, s[i].score);
}
fclose(fp);
}
int main()
{
struct stu stu_arr[MAX_STU_NUM];
for(int i=0; i<MAX_STU_NUM; i++) {
stdinForStu(&stu_arr[i]);
}
writeFileForStu(stu_arr, "student.txt");
return 0;
}
```
首先,我们定义了一个结构体`stu`,然后在`stdinForStu`函数中,通过scanf函数从键盘读入学生信息,存储到指定的结构体指针中。
在`writeFileForStu`函数中,我们打开指定文件,将学生信息按照指定格式写入到文件中,最后关闭文件。
在`main`函数中,我们创建了一个包含两名学生信息的数组,通过调用`stdinForStu`函数录入学生信息,然后再调用`writeFileForStu`函数将学生信息写入到文件中。
希望这个代码能对你有所帮助!
相关推荐
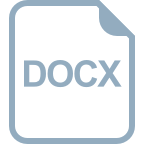
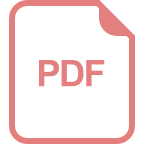
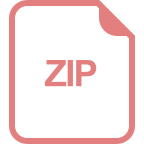














