现有一个学生表,学生信息包括:学号,姓名,性别,年龄,表中包含学生数,已经按照学号以小到大序,现在输入一个新的学生信息,然后将它插入到表中的对应位置,保证表中数据仍然按照升序排列,打印插入以后的数据表。结构体定义如下: typedef struct Studentf int sno; char name[10]: char sex;int age; Student; typedef struct Table{Student stu[10001:int num;} Table;输入多行数据第一行输入n,表示人数后面n行表示n个学生的信息,格式是:学号、姓名、性别、年龄,按升序排列接下来输入要插入的学生信息,格式是:学号、姓名、性别、年龄10输出插入后的学生信息,按升序排列
时间: 2024-02-15 12:01:45 浏览: 77
好的,我了解了你的问题。这是一个比较简单的数据结构问题,可以通过遍历查找学号位置并插入新学生信息来实现。以下是一个示例代码,你可以参考一下:
```c
#include <stdio.h>
#include <string.h>
typedef struct Student {
int sno;
char name[10];
char sex;
int age;
} Student;
typedef struct Table {
Student stu[10001];
int num;
} Table;
int main() {
Table table;
int n, i, j, k;
scanf("%d", &n);
for (i = 0; i < n; i++) {
scanf("%d %s %c %d", &table.stu[i].sno, table.stu[i].name, &table.stu[i].sex, &table.stu[i].age);
}
table.num = n;
Student newStu;
scanf("%d %s %c %d", &newStu.sno, newStu.name, &newStu.sex, &newStu.age);
// 遍历查找插入位置
for (i = 0; i < table.num; i++) {
if (newStu.sno < table.stu[i].sno) {
// 找到插入位置,后面的学生信息依次后移
for (j = table.num - 1; j >= i; j--) {
table.stu[j + 1] = table.stu[j];
}
// 插入新学生信息
table.stu[i] = newStu;
table.num++;
break;
}
}
// 遍历打印学生信息
for (i = 0; i < table.num; i++) {
printf("%d %s %c %d\n", table.stu[i].sno, table.stu[i].name, table.stu[i].sex, table.stu[i].age);
}
return 0;
}
```
以上代码中,我们定义了两个结构体,分别是`Student`和`Table`,用来存储学生和学生表的信息。在主函数中,我们先读入已有的学生信息,并将学生表的数量`num`设置为`n`。然后,我们读入新的学生信息`newStu`,并遍历查找插入位置,将插入位置后面的学生信息依次后移,最后将新学生信息插入到表中。最后,我们再次遍历学生表,打印出所有学生信息。
注意:本代码中的学生表已经按照学号升序排列,因此我们可以直接遍历查找插入位置。如果学生表没有按照学号排序,我们需要先对学生表进行排序,然后再执行插入操作。
阅读全文
相关推荐
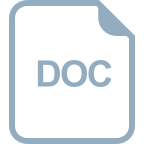
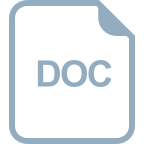
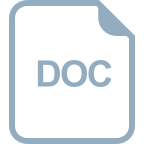
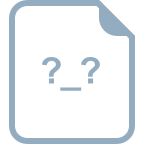
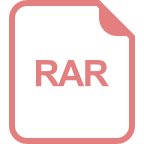



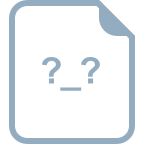









