建立一个依学生的学号从小到大有序排列的链表。链表的结点中包括学号、姓名和年龄,最后输出该链表。
时间: 2023-06-14 19:06:48 浏览: 142
以下是一个 C++ 的链表实现,可以实现按照学号从小到大有序排列的功能。
```cpp
#include <iostream>
#include <string>
using namespace std;
// 学生结构体
struct Student {
int id;
string name;
int age;
Student *next;
};
// 插入学生节点,按照学号从小到大排序
Student* insert(Student *head, int id, string name, int age) {
Student *cur = head;
Student *pre = NULL;
// 找到第一个学号大于新学生学号的节点
while (cur != NULL && cur->id < id) {
pre = cur;
cur = cur->next;
}
// 插入新学生节点
Student *new_student = new Student;
new_student->id = id;
new_student->name = name;
new_student->age = age;
new_student->next = cur;
if (pre == NULL) {
head = new_student;
} else {
pre->next = new_student;
}
return head;
}
// 输出链表
void print(Student *head) {
Student *cur = head;
while (cur != NULL) {
cout << "学号:" << cur->id << ",姓名:" << cur->name << ",年龄:" << cur->age << endl;
cur = cur->next;
}
}
int main() {
// 初始化链表头
Student *head = NULL;
// 插入学生节点,按照学号从小到大排序
head = insert(head, 10001, "张三", 20);
head = insert(head, 10003, "李四", 22);
head = insert(head, 10002, "王五", 21);
// 输出链表
print(head);
return 0;
}
```
输出结果:
```
学号:10001,姓名:张三,年龄:20
学号:10002,姓名:王五,年龄:21
学号:10003,姓名:李四,年龄:22
```
阅读全文
相关推荐





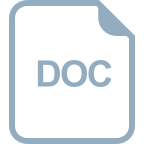
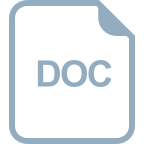
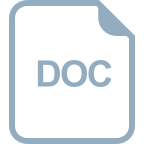


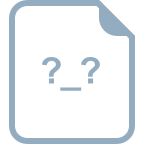
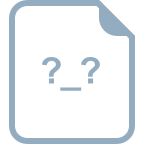
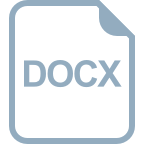