建立一个链表,每个结点包括学号、姓名、性别和出生日期,保存5名学生信息,链表按学号从小到大排序,键盘接受一名新生的信息,插入在恰当位置
时间: 2024-05-28 10:14:52 浏览: 86
好的,这个问题属于编程问题,可以回答。
以下是建立链表的程序示例:
#include <stdio.h>
#include <stdlib.h>
typedef struct student {
int id; // 学号
char name[20]; // 姓名
char gender[5]; // 性别
char birth[20]; // 出生日期
struct student *next; // 指向下一个节点的指针
} Student;
// 在链表中插入新节点
void insert_node(Student *head, Student *new_node) {
Student *p = head;
while (p->next != NULL && p->next->id < new_node->id) {
p = p->next;
}
new_node->next = p->next;
p->next = new_node;
}
// 打印链表中所有节点的信息
void print_list(Student *head) {
Student *p = head->next;
while (p != NULL) {
printf("学号:%d\t姓名:%s\t性别:%s\t出生日期:%s\n",
p->id, p->name, p->gender, p->birth);
p = p->next;
}
}
int main() {
// 初始化头节点和5个学生信息节点
Student *head = (Student *)malloc(sizeof(Student));
head->next = NULL;
Student *stu1 = (Student *)malloc(sizeof(Student));
stu1->id = 1;
strcpy(stu1->name, "张三");
strcpy(stu1->gender, "男");
strcpy(stu1->birth, "1999-01-01");
stu1->next = NULL;
Student *stu2 = (Student *)malloc(sizeof(Student));
stu2->id = 3;
strcpy(stu2->name, "李四");
strcpy(stu2->gender, "女");
strcpy(stu2->birth, "1999-02-01");
stu2->next = NULL;
Student *stu3 = (Student *)malloc(sizeof(Student));
stu3->id = 5;
strcpy(stu3->name, "王五");
strcpy(stu3->gender, "男");
strcpy(stu3->birth, "1999-03-01");
stu3->next = NULL;
Student *stu4 = (Student *)malloc(sizeof(Student));
stu4->id = 7;
strcpy(stu4->name, "赵六");
strcpy(stu4->gender, "女");
strcpy(stu4->birth, "1999-04-01");
stu4->next = NULL;
Student *stu5 = (Student *)malloc(sizeof(Student));
stu5->id = 9;
strcpy(stu5->name, "刘七");
strcpy(stu5->gender, "男");
strcpy(stu5->birth, "1999-05-01");
stu5->next = NULL;
// 将5个学生信息节点插入链表
insert_node(head, stu1);
insert_node(head, stu2);
insert_node(head, stu3);
insert_node(head, stu4);
insert_node(head, stu5);
// 键盘输入新生信息节点
Student *new_node = (Student *)malloc(sizeof(Student));
printf("请输入新生信息(学号、姓名、性别、出生日期,以空格隔开):");
scanf("%d %s %s %s", &new_node->id, new_node->name, new_node->gender, new_node->birth);
// 将新生信息节点插入链表
insert_node(head, new_node);
// 打印链表中所有节点的信息
print_list(head);
return 0;
}
阅读全文
相关推荐
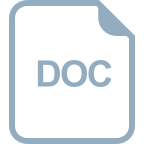
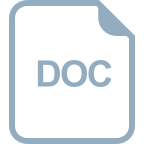
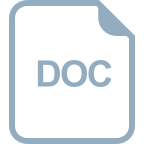








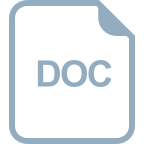
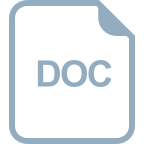
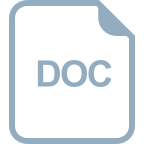
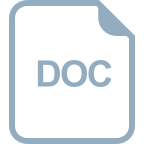
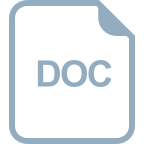
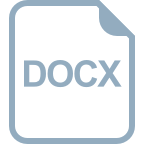