建立一个学生信息链表,包括学号,姓名,成绩,实现链表的建立C语言
时间: 2024-06-20 20:01:00 浏览: 146
在C语言中,我们可以使用结构体(struct)和链表数据结构来创建一个存储学生信息的学生链表。这里是一个简单的例子,首先定义一个`Student`结构体,然后创建一个链表节点,并实现链表的基本操作,如添加新节点、遍历等。
```c
// 定义学生结构体
typedef struct {
int student_id; // 学号
char name[50]; // 姓名
float score; // 成绩
} Student;
// 链表节点定义
typedef struct Node {
Student info;
struct Node* next;
} Node;
// 初始化链表头指针
Node* head = NULL;
// 添加新节点到链表
void addStudent(Student new_student) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->info = new_student;
newNode->next = head;
head = newNode;
}
// 添加新节点的辅助函数(已知学号插入)
void insertAtStudentId(int id, Student new_student) {
Node* current = head;
while (current && current->info.student_id != id) {
current = current->next;
}
if (current) {
newNode = (Node*)malloc(sizeof(Node));
newNode->info = new_student;
newNode->next = current->next;
current->next = newNode;
} else {
printf("Student with ID %d not found.\n", id);
}
}
// 遍历链表并打印学生信息
void printStudentList() {
Node* temp = head;
while (temp) {
printf("ID: %d, Name: %s, Score: %.2f\n",
temp->info.student_id, temp->info.name, temp->info.score);
temp = temp->next;
}
}
// 示例:创建并添加新学生
int main() {
Student new_student = {1001, "Alice", 90.5};
addStudent(new_student);
// 插入新学生到特定学号位置
insertAtStudentId(1002, {1002, "Bob", 85.0});
// 打印链表中的所有学生信息
printStudentList();
return 0;
}
阅读全文
相关推荐
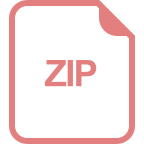

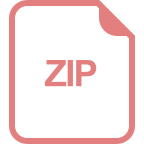













