python代码 实现A_excel中某列ip地址在另外B_excel的ip段中进行查询,返B_excel的指定列内容到A_excel中去
时间: 2023-09-04 18:04:15 浏览: 82
### 回答1:
您可以使用Python的第三方库如pandas和openpyxl来实现您的需求。
首先,您需要使用pandas读取两个Excel文件,然后在A_excel中的某列ip地址列上使用pandas的merge方法与B_excel的ip段列进行合并,合并的方式可以是内连接,如下代码:
```
import pandas as pd
# 读取A_excel文件
df_a = pd.read_excel("A_excel.xlsx")
# 读取B_excel文件
df_b = pd.read_excel("B_excel.xlsx")
# 将A_excel中的某列与B_excel中的ip段列进行合并
merged_df = pd.merge(df_a, df_b, on='ip地址列名', how='inner')
```
然后您可以使用merged_df中的数据来访问指定列的内容,并将其返回到A_excel中的指定列。下面的代码假设您要将B_excel的指定列的内容返回到A_excel的第四列:
```
# 获取B_excel中的指定列的内容
result = merged_df['指定列名']
# 将结果写入A_excel的第四列
df_a.insert(3, "第四列名", result)
# 保存A_excel
df_a.to_excel("A_excel.xlsx", index=False)
```
希望这些代码能帮助您解决问题!
### 回答2:
实现上述功能,可以使用Python的pandas库进行处理。具体步骤如下:
1. 导入pandas库,读取A_excel和B_excel的数据为两个DataFrame对象:
```python
import pandas as pd
A_data = pd.read_excel('A_excel.xlsx')
B_data = pd.read_excel('B_excel.xlsx')
```
2. 提取A_excel中ip地址列和B_excel中ip段的起始地址和终止地址列。这里假设A_excel的ip地址列为'IP',B_excel的ip段开始地址列为'StartIP',结束地址列为'EndIP':
```python
A_ip = A_data['IP']
B_start_ip = B_data['StartIP']
B_end_ip = B_data['EndIP']
```
3. 遍历A_excel中的每个ip地址,将其与B_excel中的ip段进行匹配。如果匹配成功,提取B_excel中指定列的对应内容,并将其添加到A_excel中的新列中。假设要提取B_excel的指定列为'Content',添加到A_excel的新列为'NewColumn':
```python
new_column = []
for ip in A_ip:
matched_content = ''
for i in range(len(B_data)):
if B_start_ip[i] <= ip <= B_end_ip[i]:
matched_content = B_data.at[i, 'Content']
break
new_column.append(matched_content)
A_data['NewColumn'] = new_column
```
4. 最后,将修改后的A_excel保存为新的文件:
```python
A_data.to_excel('A_excel_updated.xlsx', index=False)
```
以上就是使用Python实现将A_excel中某列ip地址在另外B_excel的ip段中进行查询,并将B_excel的指定列内容返还到A_excel中的具体代码。
### 回答3:
可以使用Python的pandas库来实现这个功能。
首先,我们需要导入pandas库,并读取A_excel和B_excel的数据。
```python
import pandas as pd
# 读取A_excel和B_excel的数据
A_data = pd.read_excel("A_excel.xlsx")
B_data = pd.read_excel("B_excel.xlsx")
```
接下来,我们需要定义一个函数来查询B_excel中的IP地址段。
```python
def search_ip(ip):
for index, row in B_data.iterrows():
start_ip = row['起始IP']
end_ip = row['结束IP']
if start_ip <= ip <= end_ip:
return row['指定列内容']
return None
```
然后,我们可以使用pandas的apply函数来对A_excel中的每个IP地址进行查询,并将查询结果填充到新的列中。
```python
A_data['查询结果'] = A_data['IP地址列'].apply(search_ip)
```
最后,我们可以将结果保存到新的Excel文件中。
```python
A_data.to_excel("查询结果.xlsx", index=False)
```
完整的代码如下所示:
```python
import pandas as pd
# 读取A_excel和B_excel的数据
A_data = pd.read_excel("A_excel.xlsx")
B_data = pd.read_excel("B_excel.xlsx")
# 定义查询函数
def search_ip(ip):
for index, row in B_data.iterrows():
start_ip = row['起始IP']
end_ip = row['结束IP']
if start_ip <= ip <= end_ip:
return row['指定列内容']
return None
# 对A_excel中的IP地址列进行查询
A_data['查询结果'] = A_data['IP地址列'].apply(search_ip)
# 保存查询结果到新的Excel文件中
A_data.to_excel("查询结果.xlsx", index=False)
```
这样,我们就实现了将B_excel的指定列内容根据IP地址段查询到A_excel中的功能。
相关推荐
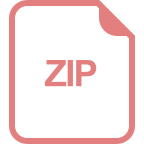
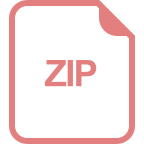














