根据已知代码写一份带表头结点单链表的排序函数代码
时间: 2024-10-04 13:04:12 浏览: 6
在C++中,我们可以创建一个带表头节点的单链表并实现排序功能。这里假设我们有一个自定义的链表节点结构(`ListNode`)和一个辅助函数(如比较函数或快速排序)。以下是一个基于插入排序的例子,它会保持表头节点不变:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点结构
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
// 自定义比较函数(根据需求修改)
bool compare(const ListNode* a, const ListNode* b) {
return a->val < b->val;
}
// 插入排序实现
void insertionSortList(ListNode*& head) {
if (head == nullptr || head->next == nullptr) {
return; // 基准情况:空链表或只有一个元素
}
ListNode* current = head->next;
head->next = nullptr; // 首先将链表断开
// 用插入排序对链表进行排序
while (current != nullptr) {
ListNode* temp = current;
while (temp->next != nullptr && compare(temp, temp->next)) {
temp = temp->next;
}
// 将temp移到其正确的位置
if (temp != current) {
temp->next = current->next;
current->next = temp;
} else {
current = current->next;
}
}
// 连接排序后的链表
current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = temp;
}
// 测试示例
int main() {
// 创建一个链表并初始化
ListNode* head = new ListNode(5);
head->next = new ListNode(3);
head->next->next = new ListNode(8);
head->next->next->next = new ListNode(1);
cout << "Original list: ";
printList(head); // 自定义打印链表的方法
insertionSortList(head);
cout << "\nSorted list: ";
printList(head);
return 0;
}
// 打印链表辅助函数
void printList(ListNode* node) {
while (node != nullptr) {
cout << node->val << " -> ";
node = node->next;
}
cout << "nullptr" << endl;
}
```
在这个例子中,`insertionSortList`函数首先将链表分为两部分:已排序的部分和未排序的部分,然后逐步将每个节点插入到正确的位置。注意,这只是一个基本的插入排序实现,对于大规模数据可能效率不高。
如果你想要其他类型的排序算法,例如快速排序、归并排序等,可以替换`compare`函数和插入排序逻辑相应部分。
相关推荐
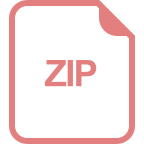
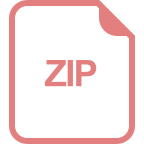














