有一个顺序表数据元素为整型数据,请设计c语言算法找出最小值,并在其后插入值为value的元素
时间: 2024-11-18 09:19:12 浏览: 21
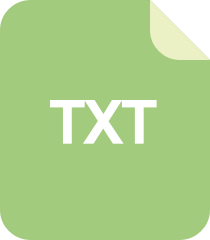
数据结构 顺序表的插入与删除算法的C语言实现

当你需要在顺序列表(数组)中找到最小值并插入新值时,可以采用以下步骤:
1. **初始化**:
- 定义两个指针 `min_index` 和 `current`,分别用于记录当前找到的最小值的位置以及遍历过程中的当前元素位置。
2. **寻找最小值**:
- 遍历整个列表(从第一个元素开始):
- 如果 `current` 指向的第一个元素比之前找到的最小值小(如果 `min_index` 未初始化,则二者相等),则更新 `min_index` 为0;否则,将 `min_index` 加一。
- 移动 `current` 指针到下一个元素。
3. **插入新值**:
- 插入操作在 `min_index+1` 的位置进行,将 `current` 指向的元素(即最小值)移动一位。
- 将 `value` 赋给新的空位。
- 更新 `current` 指针指向新插入的 `value`。
4. **结束遍历**:
- 当 `current` 指针到达数组结尾,表示所有元素已遍历完,此时 `min_index` 就是指向了原数组中最小值的位置。
5. **返回结果**:
- 最终,`array[min_index]` 就是原来的最小值,`array[min_index+1]` 存储了插入的新值 `value`。
下面是这个算法的伪代码形式:
```c
void insertAfterMin(int array[], int size, int value) {
if (size == 0) { // 如果数组为空
array[0] = value;
} else {
int min_index = 0, current = 1;
while (current < size) {
if (array[current] < array[min_index]) {
min_index = current;
}
current++;
}
// 移动元素以腾出空间
for (int i = size; i > min_index; i--) {
array[i] = array[i - 1];
}
array[min_index] = value;
}
}
```
阅读全文
相关推荐
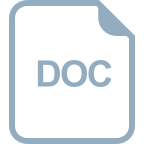
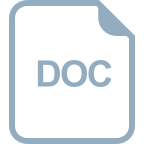


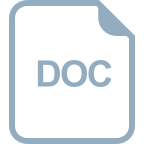
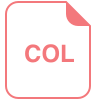
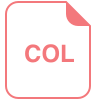
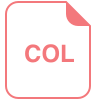
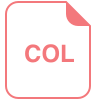
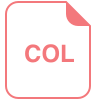
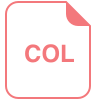
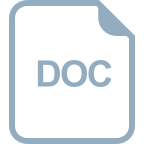
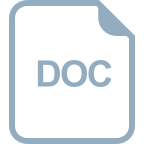
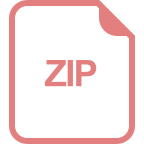
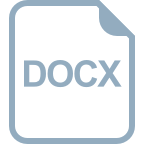